Exploring Bash Scripting: Understanding Key Flags
Bash scripting is a powerful and versatile tool that empowers users to automate tasks and streamline operations within a Unix-like environment. One crucial aspect of Bash scripting is the utilization of "flags" or command-line options, which enhance the script's flexibility and adaptability.
Flags in Bash scripts are command-line options or switches that modify the behavior of the script when it is executed. These flags, distinguished as short flags (prefixed with a single hyphen -
) or long flags (prefixed with two hyphens --), provide users with a means to customize script execution.
Whether providing input parameters, toggling features, or specifying options, flags enable a more dynamic interaction with the script. A solid understanding of these flags is essential for harnessing the full potential of Bash scripts, making them adaptable, user-friendly, and more effective tools.
Through this article we will take a look at some of the common types of flags in bash scripting and then move onto more specific flags dealing with error handling.
Table Of Contents:-
- What is Bash Scripting?
- What are Flags?
- Types Of Flags
- Errors in Bash Scripting
- Error-handling Flags
- Importance of Understanding Flags
What is Bash Scripting?
Bash scripting refers to the process of writing and executing scripts using the Bash shell, which is a command-line interpreter for Unix-like operating systems. Bash is the default shell for many Linux distributions and is also available for macOS and other Unix-like systems.
Here are some key aspects of Bash scripting and why it is important:
- Bash scripting allows users to automate repetitive tasks and processes. This can save time and reduce the risk of human error, especially for complex or lengthy sequences of commands.
- System administrators use Bash scripts to manage and configure systems. This includes tasks such as backups, log rotation, user management, and system monitoring.
- Bash scripts provide a way to customize and extend the functionality of the shell. Users can create their own commands, aliases, and functions to streamline their workflow.
- Bash scripting introduces basic programming concepts such as variables, control structures (if statements, loops), functions, and error handling. This makes it a good starting point for learning programming.
- In server environments, Bash scripts are often used for tasks like deploying applications, managing server configurations, and handling backups. Continuous Integration (CI) and Continuous Deployment (CD) pipelines frequently involve Bash scripts.
- Many CLI tools and utilities in Unix-like systems provide options for scripting and automation. Bash scripts can be used to orchestrate the execution of these tools, making it easier to integrate them into workflows.
- Bash scripts can be written to be relatively platform-independent. While Bash itself is primarily associated with Unix-like systems, it can also run on Windows using tools like Cygwin or the Windows Subsystem for Linux (WSL).
What are Flags?
In the context of a Bash script, "flags" usually refer to command-line options or switches that can be passed to the script when it is executed. These flags modify the behavior of the script, allowing users to customize its operation. Flags are commonly used to provide input parameters, toggle certain features, or specify options.
Flags are typically preceded by a hyphen (-) or a double hyphen (--). They are short, one or two-letter codes that provide additional information to the script or command. Flags in Bash scripts typically follow a specific syntax. There are two main types of flags:
Short Flags:
Short flags are usually represented by a single character and are prefixed with a single hyphen (-). Multiple short flags can be combined together.
Example:
# Usage with short flags
./myscript.sh -a -b -c
In this example, -a
, -b
, and -c
are short flags.
Long Flags:
Long flags are more descriptive and are often represented by whole words or phrases. They are prefixed with two hyphens (--).
Example:
# Usage with long flags
./myscript.sh --verbose --output-file=output.txt
Here, --verbose
and --output-file=output.txt
are long flags.
Flags are typically processed in a Bash script using constructs like getopts or by manually parsing the command-line arguments.
Here's a simple example using getopts in a Bash script:
#!/bin/bash
while getopts ":a:bf:" opt; do
case $opt in
a)
arg_a="$OPTARG"
echo "Option -a with argument: $arg_a"
;;
b)
flag_b=true
echo "Option -b is present"
;;
f)
output_file="$OPTARG"
echo "Option -f with argument: $output_file"
;;
\?)
echo "Invalid option: -$OPTARG"
exit 1
;;
:)
echo "Option -$OPTARG requires an argument."
exit 1
;;
esac
done
In this script, the getopts loop processes each option, and the corresponding action is taken based on the option and its argument (if any). You can then run the script with various flags:
./myscript.sh -a value -bf -f output.txt
This script would output:
Option -a
with argument: value
Option -b
is present
Option -f
with argument: output.txt
Types of Flags
i.) Single-letter flags:
Single-letter flags are often preceded by a single hyphen (-) and can be combined. For example, -a
-b
-c
can be written as -abc
.
# Example with single-letter flags
script.sh -a -b -c
ii.) Long flags:
Long flags are often preceded by two hyphens (--). They are more descriptive than single-letter flags.
# Example with long flags
script.sh --verbose --output-file=output.txt
iii.) Combining short and long flags:
You can combine short and long flags in a single command.
# Example with short and long flags
script.sh -abc --verbose --output-file=output.txt
iv.) Flag arguments:
Some flags may require additional arguments. These arguments are typically provided after the flag.
# Example with a flag and its argument
script.sh --input-file input.txt
In the script, you can access the flag arguments using special variables like $1, $2, etc., where $1 represents the first argument, $2 represents the second argument, and so on.
v.) Boolean flags:
Some flags are Boolean and don't require additional arguments. Their presence indicates a true or false condition.
# Example with a boolean flag
script.sh --force
In the script, you can check if a Boolean flag is present by testing its existence.
Here's a simple example of a Bash script that uses flags:
#!/bin/bash
while [[ $# -gt 0 ]]; do
case "$1" in
-a|--apple)
echo "Option -a or --apple is present."
;;
-b|--banana)
echo "Option -b or --banana is present."
;;
-c|--count)
if [[ -n "$2" ]]; then
count="$2"
echo "Count is set to $count."
shift
else
echo "Error: Count requires a value."
exit 1
fi
;;
*)
echo "Unknown option: $1"
exit 1
;;
esac
shift
done
In this example, the script uses a while
loop and a case
statement to handle different flags. The shift command is used to move through the list of arguments.
You can run this script with commands like:
./myscript.sh -a -b --count 5
This would output:
Option -a
or --apple
is present.
Option -b
or --banana
is present.
Count is set to 5.
Errors in Bash Scripting
Bash scripts, like any other programs, can encounter various types of errors.
i. ) Syntax errors occur when there is a mistake in the script's syntax, such as a missing or misplaced character, incorrect command structure, or misuse of shell constructs.
# Syntax error: missing closing parenthesis
if [ -f "file.txt" then
echo "File exists."
fi
ii.) Runtime errors occur during the execution of the script. These errors can be caused by various issues, such as attempting to perform an operation on an undefined variable or calling a command that does not exist.
# Runtime error: variable not defined
echo "The value of variable is: $undefined_variable"
iii.) Logical errors are bugs in the script's logic that lead to unexpected behavior. The script may run without syntax errors, but it does not produce the intended results.
# Logical error: incorrect condition
if [ $variable -eq 0 ]; then
echo "Variable is equal to 0."
else
echo "Variable is not equal to 0." # Logical error
fi
iv.) Bash commands and scripts return an exit status to indicate success or failure. An exit status of 0 typically indicates success, while a non-zero value signifies an error. Failure to check and handle non-zero exit statuses can lead to issues.
# Exit status error: command not found
non_existent_command
echo "Command executed successfully."
v.) Failing to validate user input or command-line arguments can lead to unexpected behavior. It's essential to ensure that inputs are of the expected type and within acceptable ranges.
# Input validation error: expecting a number
read -p "Enter a number: " user_input
echo "You entered: $user_input"
Handling errors in Bash scripts often involves implementing proper error-checking mechanisms, using conditional statements to verify the success of commands, and employing techniques like set -o errexit to make the script exit on the first error. Additionally, logging error messages and providing meaningful feedback to users can aid in debugging and troubleshooting.
Error-handling Flags
1. set -o errexit or set -e:
This option makes the script exit immediately if any command it runs exits with a non-zero status (i.e., an error). It helps in making scripts more robust by ensuring that errors are not ignored, and the script stops execution if any command fails.
Example:
#!/bin/bash
set -e
echo "This is a command that fails"
command_that_fails # This will cause the script to exit immediately
echo "This line will not be executed"
2. set -o nounset or set -u:
This option treats unset variables as errors. If the script tries to use a variable that has not been set (assigned a value), the script will exit.
Example:
#!/bin/bash
set -u
unset_variable="$undefined_variable" # This will cause the script to exit immediately
echo "This line will not be executed"
3. set -o pipefail:
By default, a pipeline in a Bash script returns the exit status of the last command in the pipeline. If any command in the pipeline fails, the entire pipeline is considered to have failed, even if other commands succeed.
When set -o pipefail
is used, the pipeline returns the exit status of the last (rightmost) command to exit with a non-zero status, or zero if all commands exit successfully. This can be useful for more accurately determining the success or failure of a pipeline.
Example:
#!/bin/bash
set -o pipefail
command1 | command2 | command3
if [ $? -eq 0 ]; then
echo "Pipeline succeeded"
else
echo "Pipeline failed"
fi
Importance of Understanding Flags
Understanding flags in Bash scripting is crucial for several reasons. Firstly, it allows users to tailor the script's behavior to suit specific requirements, enhancing the script's adaptability in various scenarios. Flags provide a mechanism for users to input information, toggle features on or off, and control the flow of the script's execution.
Secondly, a comprehensive grasp of flags facilitates effective communication between the user and the script. By providing a clear and concise interface for customization, flags enhance the user experience and make scripts more user-friendly. This is particularly important in scenarios where scripts are shared or used by individuals with varying requirements.
Moreover, the ability to work with flags introduces users to fundamental scripting concepts, such as parsing command-line arguments, handling options, and implementing conditional logic. This not only contributes to a deeper understanding of Bash scripting but also serves as a foundational skill for broader programming endeavors.
Conclusion
Flags in Bash scripts provide a convenient way to make scripts more flexible and versatile, allowing users to tailor the script's behavior according to their needs.
However, it's essential to use them judiciously, as in some cases, you may want to handle errors differently or continue execution despite errors, depending on the specific requirements of your script.
Learning Bash scripting is a valuable skill for system administrators, developers, and anyone who interacts with the command line in their daily work.
Infrastructure Monitoring with Atatus
Track the availability of the servers, hosts, virtual machines and containers with the help of Atatus Infrastructure Monitoring. It allows you to monitor, quickly pinpoint and fix the issues of your entire infrastructure.
In order to ensure that your infrastructure is running smoothly and efficiently, it is important to monitor it regularly. By doing so, you can identify and resolve issues before they cause downtime or impact your business.
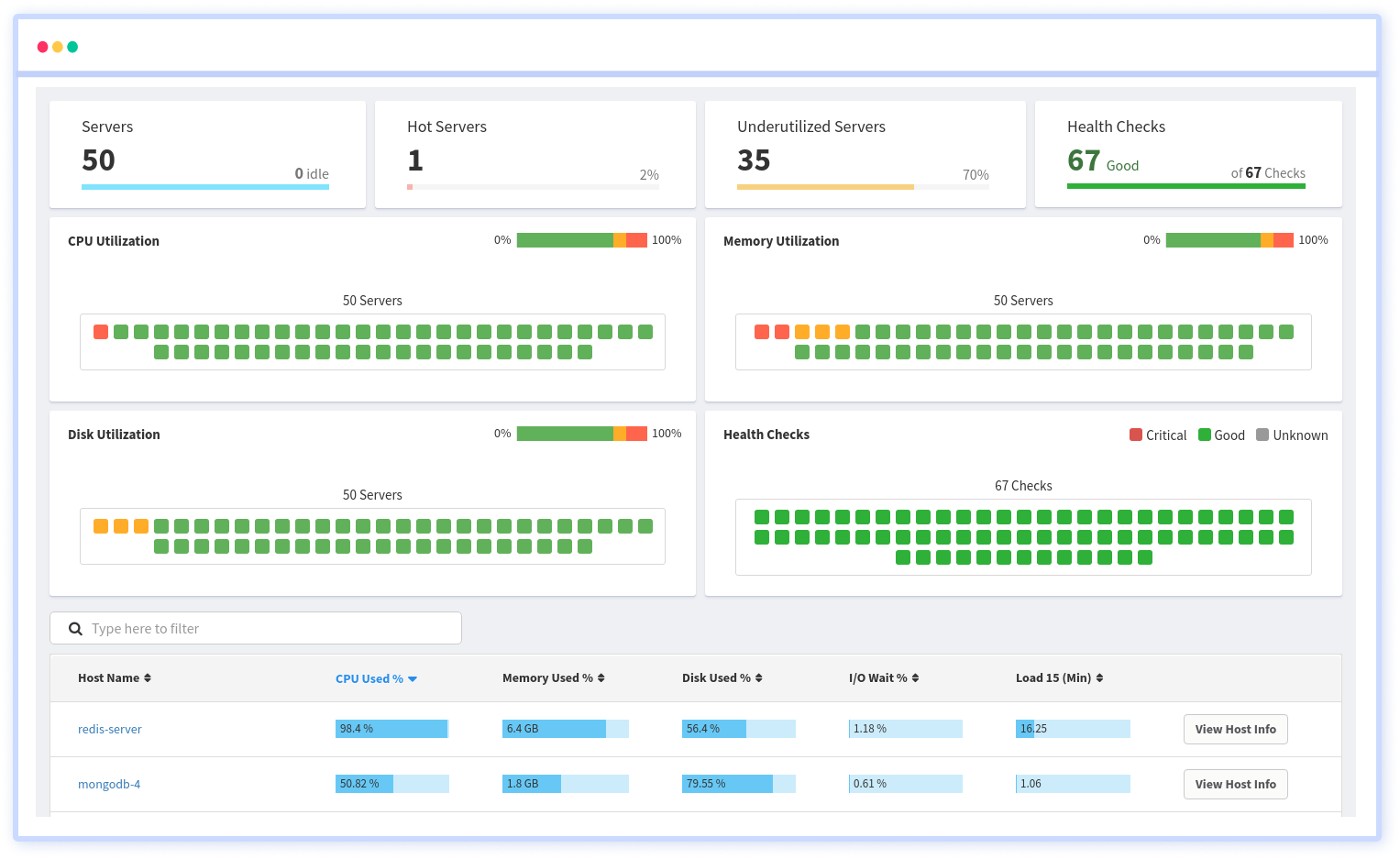
It is possible to determine the host, container, or other backend component that failed or experienced latency during an incident by using an infrastructure monitoring tool. In the event of an outage, engineers can identify which hosts or containers caused the problem. As a result, support tickets can be resolved more quickly and problems can be addressed more efficiently.
Start your free trial with Atatus. No credit card required.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More