Essential Guide to HTTP POST Request Method
The POST method is a crucial part of the HTTP protocol that allows data submission from clients to servers. Unlike other methods like GET, POST sends data in the request body, making it ideal for secure and confidential information.
By understanding the POST method, you can enable powerful functionalities such as form submissions, resource creation, and API operations.
One of the distinguishing features of the POST method is its ability to handle sensitive and confidential data. Since the data is transmitted in the request body rather than in the URL, it offers a more secure approach for transmitting information such as passwords, personal details, or financial data.
Let us explore the ins and outs of the POST method in web development. We'll uncover its abilities, understand how it works, and discover how it can enhance your web development projects.
Table of Contents
- POST Request Method
- Components of a POST Request Method
- Sending HTTP Headers with HTTP POST Request
- Use cases of POST Method
- POST Method VS GET Method
- Key Points to Remember
POST Request Method
In web development, when you want to send data from a client (such as a web browser) to a server, you can use different HTTP methods. One of these methods is called a POST request.
A POST request is used when you want to submit data to the server to create or update a resource.
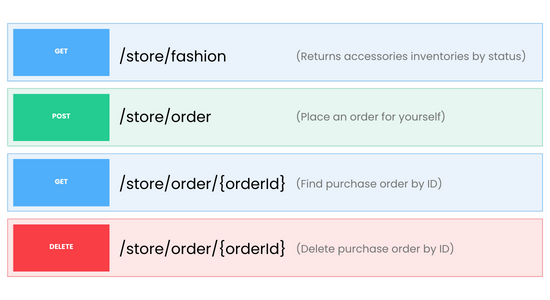
For example, let's say you have a simple website with a form where users can submit their email addresses to subscribe to a newsletter. When a user enters their email address and clicks the submit button, the form will typically send a POST request to the server.
Here's a simple example to illustrate how a POST request works. Let's assume you have a web page with a form like this,
<form action="/subscribe" method="post">
<input type="email" name="email" placeholder="Enter your email">
<button type="submit">Subscribe</button>
</form>
In this example, When a user fills out an HTML form and clicks the submit button, the browser sends a POST request to the server at the specified URL, which is mentioned in the form's action attribute.
In this case, the URL is "/subscribe". The POST request carries the data entered in the form, such as the email address, within its body.
On the server-side, the server receives this POST request and extracts the data from the request's body. It can then perform various actions with the data, such as storing the email address in a database, sending a confirmation email to the user, or processing the information in any other required way.
The POST request method is commonly used when creating or updating resources on the server, allowing users to submit data and interact with web applications.
Components of a POST Request Method
A POST request involves several key components that play important roles in transmitting data from a client to a server. Understanding these components is crucial for effectively utilizing and implementing POST requests in web development. Let us take a look at each of these components:
- URL/Endpoint: The URL (Uniform Resource Locator) or endpoint specifies the server location where the POST request is sent. It identifies the specific resource or action that the server should handle. It acts as a unique address for accessing a particular web page, service, or API endpoint.
- Request Method: The request method indicates the type of action being performed on the server. In the case of a POST request, it signifies that data is being submitted to the server to create or update a resource. Other common request methods include GET (retrieving data), PUT (updating data), and DELETE (removing data).
- Request Header: The request header contains additional information about the request. It includes metadata and instructions for the server, such as the content type being sent, character encoding, authentication credentials, and details related to caching, language preferences, or user agents.
- Request Body: The request body carries the actual data being sent in the POST request. It contains the payload or content that needs to be processed by the server. This can include form data, JSON, XML, or other structured data formats. The request body is where the relevant data is placed for the server to process and take appropriate actions.
- Response: The response is the server's reply to the client's request. It contains the result of the requested action, including status information, data from the server (if applicable), and additional metadata. The response allows the client to understand the outcome of the request and handle it accordingly.
- Content-Length: A header field that specifies the length or size of the request body in bytes. It helps the server know the size of the incoming data.
- Authentication: If the server requires authentication, the POST request may need to include credentials or tokens in the headers or body to authenticate the client making the request.
Here's an example that includes all the components of a POST request in a single example:
POST /api/users HTTP/1.1
Host: api.example.com
Content-Type: application/json
Authorization: Bearer <access_token>
User-Agent: MyClient/1.0
Content-Length: 64
{
"name": "John Doe",
"email": "johndoe@example.com",
"age": 30
}
In this example:
- The request method is
POST
. - The request URI is
/api/users
. - The HTTP version is
HTTP/1.1
. - The
Host
header specifies the host or domain of the server (api.example.com
in this case). - The
Content-Type
header indicates that the request body is in JSON format. - The
Authorization
header contains the access token for authentication. - The
User-Agent
header identifies the client making the request. - The
Content-Length
header specifies the length of the request body in bytes. - The request body contains the user data in JSON format.
These components together form a complete example of a POST request, which can be sent to the server to create a new user or perform other operations as per the API's specifications.
Sending HTTP Headers with HTTP POST Request
When sending an HTTP POST request, you have the option to include headers in the request. Headers provide additional information about the request or the data being sent. Here's an overview of how to send HTTP headers with an HTTP POST request:
1. Content-Type
One important header to include is the Content-Type header. It specifies the format of the data being sent in the request body. Common Content-Type values include,
- application/json: for sending JSON data
- application/x-www-form-urlencoded: for sending form data
- multipart/form-data: for sending binary or file data
Example:
Content-Type: application/json
2. Authorization
If your server requires authentication, you may need to include the Authorization header. This header typically includes a token or credentials to authenticate the request.
Example:
Authorization: Bearer your-token-here
3. Custom Headers
You can also include custom headers to convey specific information or instructions to the server. These headers can be defined by the server or the application.
Example:
X-Requested-With: XMLHttpRequest
4. Accept
The Accept header indicates the media types that the client is able to handle in the response. It specifies the preferred format for the server to send the response data back to the client.
Example:
Accept: application/json
These are just a few examples of commonly used headers with HTTP POST requests. The headers you include may vary depending on the specific requirements of the server or the desired behaviour of the request.
The headers you choose to include should align with the server's expectations and any additional functionality you wish to incorporate.
Use cases of POST Method
The POST method is commonly used in web development for various purposes. Here are some common use cases for the POST method:
1. Form Submission
When a user submits a form on a website, the data entered in the form fields is typically sent to the server using a POST request. This allows the server to process and store the submitted data.
<html>
<head>
<title>Form Submission Example</title>
</head>
<body>
<h1>Contact Form</h1>
<form action="/submit-form" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<label for="message">Message:</label><br>
<textarea id="message" name="message" rows="4" cols="50" required></textarea><br><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
In this example, we have a simple contact form with fields for name, email, and message.
2. Creating Resources
POST requests are often used to create new resources on a server. For example, when adding a new item to an online shopping cart or creating a new post in a blogging platform, the data describing the new resource is sent to the server via a POST request.
3. Uploading Files
When uploading files, such as images or documents, to a server, the POST method is used. The file data is included in the request body, allowing the server to receive and save the file on the server-side.
Here's an example of how you can upload files using the POST method in Node.js:
const axios = require('axios');
const FormData = require('form-data');
const fs = require('fs');
async function uploadFile() {
const url = 'http://example.com/upload';
const filePath = 'path/to/file.jpg';
const form = new FormData();
form.append('file', fs.createReadStream(filePath));
try {
const response = await axios.post(url, form, {
headers: form.getHeaders()
});
console.log(response.data);
} catch (error) {
console.error(error);
}
}
uploadFile();
In this example, we're using the axios
library for making the HTTP POST request and the form-data
library to handle multipart form data. We create a new FormData
instance, append the file to it using fs.createReadStream()
, and then send the POST request with the appropriate headers.
4. API Operations
POST requests are commonly used in API (Application Programming Interface) operations to perform actions that modify or create resources on the server. For example, creating a new user account, submitting a comment, or updating a database record.
Here's an example of how you can perform API operations using the POST method in Node.js:
const axios = require('axios');
async function performAPIOperation() {
const url = 'http://example.com/api/operation';
const data = {
operation: 'some_operation',
parameter1: 'value1',
parameter2: 'value2'
};
try {
const response = await axios.post(url, data);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
performAPIOperation();
Replace 'http://example.com/api/operation'
with the actual endpoint URL for performing the API operation. Adjust the data
object according to the operation and parameters required by your API.
5. Data Modification
In some cases, the POST method is used to update or modify data on the server. While this is not the standard usage (as it should ideally be done using the PUT or PATCH methods), certain systems or frameworks may handle data modification through POST requests.
Here's an example of how you can perform data modification using the POST method in Node.js:
const axios = require('axios');
async function modifyData() {
const url = 'http://example.com/api/data';
const data = {
id: 123,
name: 'Updated Name',
description: 'Updated Description'
};
try {
const response = await axios.post(url, data);
console.log(response.data);
} catch (error) {
console.error(error);
}
}
modifyData();
Replace 'http://example.com/api/data'
with the actual endpoint URL for modifying the data. Adjust the data
object according to the specific data modification requirements of your application.
POST Method VS GET Method
Parameters | POST Method | GET Method |
---|---|---|
Purpose | The POST method is primarily used to submit data from the client to the server, often for creating new resources or performing actions that modify server data. | The GET method is used to retrieve data from the server without making any modifications. It requests a representation of a resource identified by a URL. |
Row Data Handling | In a POST request, data is sent in the body of the request. It can include form data, JSON, XML, or other structured formats, allowing for larger amounts of data to be transmitted. | In a GET request, data is sent through the URL's query parameters. The data is visible in the URL and has limitations on the amount of data that can be transmitted. |
Security | POST requests are generally considered more secure as the data is transmitted in the request body, which is not directly visible in the URL. | GET requests are less secure as the data is visible in the URL, making it susceptible to being intercepted or stored in logs. |
Caching | POST requests are generally not cached by browsers or intermediate caches since they typically modify server data. | GET requests can be cached by browsers and intermediate caches since they are considered safe and do not modify server data. |
Idempotence | POST requests are not idempotent. Repeating the same POST request may result in different outcomes each time, such as creating multiple resources or triggering different actions. | GET requests are idempotent. Repeating the same GET request will produce the same result without any side effects. |
Key Points to Remember
- The POST method is used to submit data from clients to servers in web development.
- Unlike the GET method, POST sends data in the request body, allowing for secure and confidential information transmission.
- Common use cases for the POST method include form submissions, resource creation, file uploads, API operations, and data modification.
- Handle sensitive data securely by utilizing the POST method for transmission.
- Validate and process the incoming POST requests on the server-side.
- Adhere to best practices and industry standards when implementing the POST method.
- Continuously update your knowledge of POST method advancements and industry trends.
- By mastering the POST method, you can enhance user experiences and add powerful functionalities to your web applications.
Conclusion
In conclusion, the HTTP POST request method is a crucial component of web communication, facilitating the secure and efficient transmission of data from a client to a server.
By utilizing the request body, POST requests allow for the creation and modification of resources on the server, making them essential for a wide range of web applications.
The advantages of the HTTP POST method include its ability to handle large amounts of data, support for different data formats, and enhanced security measures compared to the GET method.
Its versatility makes it a fundamental tool for developers, enabling the implementation of secure user authentication and authorization systems.
By understanding and effectively utilizing the HTTP POST method, developers can build robust and interactive web applications that meet the needs of users and provide a seamless online experience.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
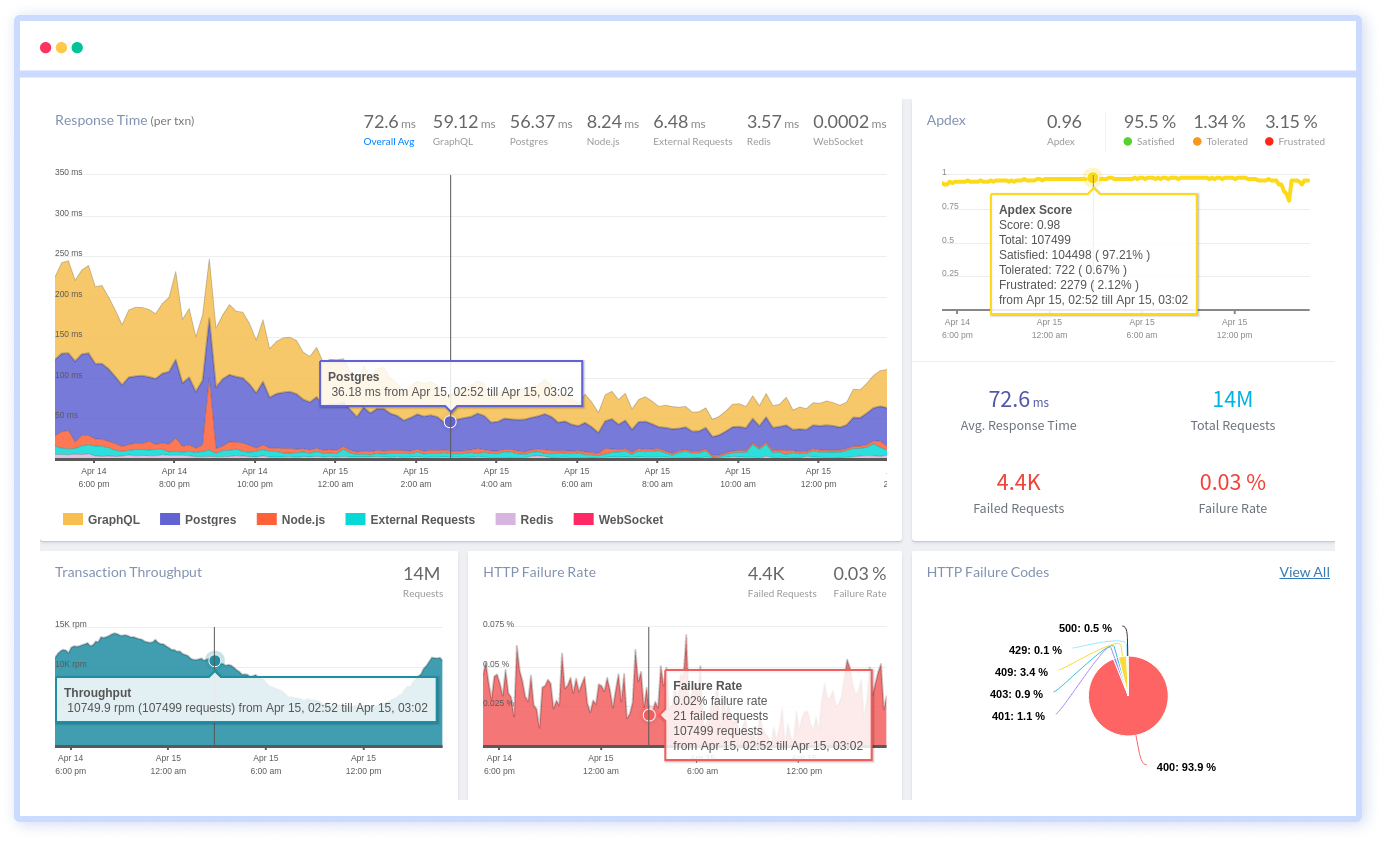
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More