Java Methods Unraveled: A Primer for Java Developers
Java is an object-oriented programming language that offers a range of powerful features for building robust and scalable applications. One of the fundamental concepts in Java is methods, which allow you to organize and encapsulate reusable code in a program.
A method in Java is a block of code that performs a specific task and can be called multiple times from within a program. It can accept input parameters and return a result, making it a versatile tool for building complex applications.
In this blog, we will explore the various aspects of methods in Java, including method declaration and syntax, parameter passing, return values, and method overloading. We'll also look at some best practices for writing effective and efficient methods in Java.
By the end of this blog, you'll have a solid understanding of how methods work in Java and how you can use them to write cleaner, more maintainable code. So let's dive in and explore the world of methods in Java!
Table of Contents
- What are Methods?
- Components in Method Declaration
- Types of Java Methods
- Parameter Passing in Java
- Return Values in Java
- Method Overloading in Java
- Use cases of Methods
What are Methods?
A method is a block of code associated with an object that performs certain tasks when it is called. It is part of a class. We can pass data into methods that are known as parameters. Methods should be created within a class.
Java also has constructor methods. A constructor is a special method that creates an object of a class.
Method Declaration in Java
As we said earlier methods should be declared within a class. The following syntax is used to declare a method in Java.
return_type method_name(parameters list){
//method body
}
For example,
public int addNumbers (int a, int b){
//method body
}
Here Public is an access modifier, int is the return type, addNumbers
is a method name, and finally, int a
& int b
are parameters passed into the method addNumber
.
How to call a method in Java?
In Java, you can call a method by using its name and passing the required arguments, if any. The basic syntax for calling a method is as follows:
object.methodName(arguments);
Here, object
is the object on which the method is being called, methodName
is the name of the method, and arguments
are the values passed to the method (if the method requires any).
For example, let's say we have a method addNumbers
in a class called Calculator
that takes two integer parameters and returns their sum:
public class Calculator {
public int addNumbers(int a, int b) {
int sum = a + b;
return sum;
}
}
To call this method, we need to create an instance of the Calculator
class and then call the method on that object:
Calculator myCalculator = new Calculator();
int result = myCalculator.addNumbers(5, 7);
In this example, we create an instance of the Calculator
class using the new
keyword, and store it in a variable called myCalculator
. We then call the addNumbers
method on this object, passing it the integer values 5
and 7
. The method returns the sum of these values, which is stored in the variable result
.
Note that if the method is declared as static
, it can be called directly on the class without creating an object. For example, if the addNumbers
method in our example was declared as static
, we could call it like this:
int result = Calculator.addNumbers(5, 7);
Here, we are calling the addNumbers
method directly on the Calculator
class, without creating an instance of the class.
Components in Method Declaration
There are 6 components included in the method declaration.
1. Method signature
Method signature is a combination of the method's name, parameter types, and the number of parameters. It does not include the return type of the method, nor does it include any modifiers such as public, private, or static. The method signature is used to uniquely identify a method in a class.
Here's an example method signature:
public int add(int x, int y)
In this example, the method signature includes the method name add
, and two integer parameters (int x, int y)
. The return type of the method is int
, but it is not included in the method signature.
It's important to note that if you have two methods with the same name but different signatures, they are considered to be different methods. This is known as method overloading. Here's an example:
public int add(int x, int y)
public int add(int x, int y, int z)
In this example, we have two methods with the same name add
, but different signatures. The first method takes two integer parameters, while the second method takes three integer parameters. Java is able to distinguish between these methods based on their unique signatures.
2. Method name
It is used to name the method. It is crucial to name your methods correctly because this will make it easier for the programmer to assess your program and for the debugging processes.
public class Main {
static void myMethod() {
// code to be executed
}
}
3. Access Modifier
It is used to specify the method's access type. There are 4 types of access modifiers in Java.
i.) private
The private access modifier in Java restricts access to a variable, method, or class to only the class where it is defined. For example, if you have a private variable in a class called "Person", only the methods within the same class can access it.
public class Person {
private String name;
private int age;
private void setName(String name) {
this.name = name;
}
private void setAge(int age) {
this.age = age;
}
}
ii.) public
The public access modifier in Java allows a variable, method, or class to be accessible from any other class or package.
For example, if you have a public method in a class called "Car", other classes can use it to modify or access the car's properties.
public class Car {
public int speed;
public void accelerate() {
speed += 10;
}
}
iii.) protected
The protected access modifier in Java allows access to a variable, method, or class to its subclasses and to the classes within the same package.
For example, if you have a protected variable in a class called "Animal", it can be accessed by its subclass "Dog".
public class Animal {
protected String name;
protected void setName(String name) {
this.name = name;
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Woof!");
}
public void setName(String name) {
super.setName(name);
}
}
iv.) default
The default access modifier in Java (also called package-private) allows access to a variable, method, or class only within the same package.
For example, if you have a default method in a class called Utils
, it can be accessed by other classes within the same package.
class Utils {
void printMessage(String message) {
System.out.println(message);
}
}
4. Return Type
It aids in determining the return value of the methods; depending on the requirements of the program, it may be an int, char, or long. If the program isn't returning any values, it may be void.
public class ReturnTypeTest1 {
public int add() { // without arguments
int x = 30;
int y = 70;
int z = x + y;
return z;
}
public static void main(String args[]) {
ReturnTypeTest1 test = new ReturnTypeTest1();
int add = test.add();
System.out.println("The sum of x and y is: " + add);
}
}
5. Parameter List
It indicates the list of arguments (data type and variable name) that will be used in the method. It is used to send input parameters from the main function to our methods. If there is no variable accessible as a parameter, methods should be encased in brackets.
public class Main {
static void myMethod(String fname, int age) {
System.out.println(fname + " is " + age);
}
public static void main(String[] args) {
myMethod("Liam", 5);
myMethod("Jenny", 8);
myMethod("Anja", 31);
}
}
Here, fname, and age are parameters. While Liam, Jenny, and Anja are arguments.
6. Method body
Method body is the part of a method that contains the statements and code that are executed when the method is called. It is enclosed in curly braces { } after the method signature, and contains the code that performs the specific action of the method.
Here is an example of a method signature and method body:
public int addNumbers(int a, int b) {
int sum = a + b;
return sum;
}
In this example, the method signature is public int addNumbers(int a, int b)
, which specifies that the method is a public method that takes two integer parameters called a
and b
, and returns an integer value.
The method body contains two statements: the first statement calculates the sum of the two integer parameters and assigns it to a new variable called sum
. The second statement uses the return
keyword to return the value of sum
to the calling method.
Types of Java Methods
In Java, there are several types of methods that can be defined. Here are some of the most common types:
1. Instance Methods
Instance methods are associated with objects of a class and can access instance variables and methods of that class. Here's an example:
public class Person {
private String name;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Person person = new Person();
person.setName("John");
System.out.println(person.getName());
2. Static methods
Static methods belong to the class itself and can be called using the class name, without creating an object of the class. Here's an example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
int sum = MathUtils.add(5, 10);
System.out.println(sum);
3. Abstract methods
Abstract methods are declared in an abstract class but do not have an implementation. The implementation is provided by the subclass that extends the abstract class. Here's an example:
public abstract class Shape {
public abstract double area();
}
public class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Shape circle = new Circle(5);
System.out.println(circle.area());
4. Final Methods
Final methods cannot be overridden by subclasses. Here's an example:
public class Vehicle {
public final void start() {
// Code to start the vehicle
}
}
public class Car extends Vehicle {
// This will result in a compilation error
public void start() {
// Code to start the car
}
}
5. Native Methods
Native methods are written in a programming language other than Java and are executed using the Java Native Interface (JNI). Here's an example:
public class HelloWorld {
public native void print();
static {
System.loadLibrary("HelloWorld");
}
public static void main(String[] args) {
HelloWorld helloWorld = new HelloWorld();
helloWorld.print();
}
}
Parameter Passing in Java
1. Pass-by-Value
In Java, the values of arguments are copied and passed to methods when they are called. This means that any modifications made to the parameters inside the method do not affect the original arguments. Therefore, the original values of the arguments remain unchanged after the method call.
public class PassByValueExample {
public static void main(String[] args) {
int num = 10;
System.out.println("Value of num before calling method: " + num);
changeValue(num);
System.out.println("Value of num after calling method: " + num);
}
public static void changeValue(int number) {
number = 20;
}
}
Output:
Value of num before calling method: 10
Value of num after calling method: 10
In the above example, we pass the num
variable to the changeValue()
method, but the value of num
remains the same after the method call. This is because number
is a copy of the original value of num
, and any changes made to number
inside the method do not affect the original num
variable.
2. Pass-by-Reference
Java does not support pass-by-reference. However, it is possible to simulate pass-by-reference by passing an object reference as an argument. In this case, a copy of the reference is passed to the method, but the object itself is not copied. Any changes made to the object inside the method will be reflected in the original object.
Here's an example:
public class PassByReferenceExample {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder("Hello");
System.out.println("Value of sb before calling method: " + sb);
changeValue(sb);
System.out.println("Value of sb after calling method: " + sb);
}
public static void changeValue(StringBuilder str) {
str.append(" World!");
}
}
Output:
Value of sb before calling method: Hello
Value of sb after calling method: Hello World!
In the above example, we pass the sb
object reference to the changeValue()
method. Inside the method, we modify the sb
object by appending " World!" to it. This change is reflected in the original sb
object outside the method.
3. Passing Primitive Data Types
Primitive data types such as int
, float
, boolean
, etc. are always passed by value in Java. Here's an example:
public class PrimitiveDataTypeExample {
public static void main(String[] args) {
int num = 10;
System.out.println("Value of num before calling method: " + num);
changeValue(num);
System.out.println("Value of num after calling method: " + num);
}
public static void changeValue(int number) {
number = 20;
}
}
Output:
Value of num before calling method: 10
Value of num after calling method: 10
4. Passing Objects
When an object is passed as an argument, the object reference is passed by value. This means that a copy of the reference to the object is passed, but not the object itself. Here's an example:
public class ObjectExample {
public static void main(String[] args) {
Person person = new Person("John", 25);
System.out.println("Person before method call: " + person);
changeValue(person);
System.out.println("Person after method call: " + person);
}
public static void changeValue(Person p) {
p.setAge(30);
}
}
Output:
Person before method call: Person [name=John, age=25]
Person after method call: Person [name=John, age=30]
Return Values in Java
1. Returning Primitive Data Types
When a method in Java returns a primitive data type, it means that the method returns a value of that data type. For example, a method that returns an int will return an integer value.
public class Example {
public static void main(String[] args) {
int sum = add(2, 3);
System.out.println(sum); // Output: 5
}
public static int add(int a, int b) {
return a + b;
}
}
In the above example, the add
method returns an int value, which is the sum of its two parameters, a
and b
. The main
method calls the add
method and assigns its return value to the variable sum
.
2. Returning Objects
In Java, a method can also return an object. An object is an instance of a class, and when a method returns an object, it returns an instance of that class.
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
public class Example {
public static void main(String[] args) {
Person person = createPerson("John");
System.out.println(person.getName()); // Output: John
}
public static Person createPerson(String name) {
return new Person(name);
}
}
In the above example, the createPerson
method returns a Person
object with the specified name. The main
method calls the createPerson
method and assigns its return value to the variable person
. Then, it calls the getName
method on the person
object to get its name.
3. Returning Multiple Values
Java does not support returning multiple values directly. However, you can use various techniques to return multiple values, such as returning an object that contains multiple values or returning an array.
public class Example {
public static void main(String[] args) {
int[] values = getValues();
System.out.println("Value 1: " + values[0]); // Output: Value 1: 1
System.out.println("Value 2: " + values[1]); // Output: Value 2: 2
}
public static int[] getValues() {
int[] values = {1, 2};
return values;
}
}
In the above example, the getValues
method returns an array of two integers. The main
method calls the getValues
method and assigns its return value to the values
variable. Then, it prints the two values in the array using their indexes.
Method Overloading in Java
Method overloading is a feature in Java that allows you to define multiple methods with the same name in the same class, as long as they have different parameter lists. When a method is called, the Java compiler determines which method to invoke based on the arguments passed to the method and the method signature.
1. Overloading by Changing Number of Argument
You can overload a method by changing the number of arguments it accepts. Here's an example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
public static int add(int a, int b, int c) {
return a + b + c;
}
}
int sum1 = MathUtils.add(5, 10);
int sum2 = MathUtils.add(5, 10, 15);
System.out.println(sum1); // Output: 15
System.out.println(sum2); // Output: 30
In the above example, we have defined two methods with the same name "add", but with different numbers of arguments. The first method takes two integer arguments, and the second method takes three integer arguments. When we call the "add" method with two arguments, the first method is called, and when we call the "add" method with three arguments, the second method is called.
2. Overloading by Changing Data Types of Arguments
You can also overload a method by changing the data types of its arguments. Here's an example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
public static double add(double a, double b) {
return a + b;
}
}
int sum1 = MathUtils.add(5, 10);
double sum2 = MathUtils.add(5.5, 10.5);
System.out.println(sum1); // Output: 15
System.out.println(sum2); // Output: 16.0
In the above example, we have defined two methods with the same name "add", but with different data types of arguments. The first method takes two integer arguments, and the second method takes two double arguments. When we call the "add" method with two integer arguments, the first method is called, and when we call the "add" method with two double arguments, the second method is called.
3. Overloading by Changing Order of Arguments
You can also overload a method by changing the order of its arguments. Here's an example:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
public static int add(int a, int b, int c) {
return a + b + c;
}
public static int add(int a, int b, int c, int d) {
return a + b + c + d;
}
}
int sum1 = MathUtils.add(5, 10);
int sum2 = MathUtils.add(5, 10, 15);
int sum3 = MathUtils.add(5, 10, 15, 20);
System.out.println(sum1); // Output: 15
System.out.println(sum2); // Output: 30
System.out.println(sum3); // Output: 50
In the above example, we have defined three methods with the same name "add", but with different orders of arguments. The first method takes two integer arguments, the second method takes three integer arguments, and the third method takes four integer arguments and returns their sum each.
This is because the first call to add
returns the sum of 5 and 10, which is 15. The second call to add
returns the sum of 5, 10, and 15, which is 30. The third call to add
returns the sum of 5, 10, 15, and 20, which is 50.
Use cases of Methods
- Reusability: Methods allow you to write code once and reuse it in multiple places. This makes your code more modular and easier to maintain.
- Abstraction: Methods allow you to hide the implementation details of a task and provide a higher-level interface for other code to use. This makes it easier to use and understand your code.
- Encapsulation: Methods allow you to encapsulate behavior and data into objects, which can then be used by other parts of your code without exposing their internal implementation.
- Code organization: Methods can help you organize your code into logical units, making it easier to read and understand.
- Modularity: Methods allow you to break down a complex task into smaller, more manageable pieces. This makes it easier to work on individual pieces of the code without affecting the rest of the program.
- Polymorphism: Methods allow you to define a single behavior that can be applied to different objects. This is known as polymorphism and is a powerful concept in object-oriented programming.
- Code reuse: Methods can be reused in other projects, as long as the classes and methods are public and accessible.
Conclusion
In conclusion, methods in Java are an essential concept in object-oriented programming that allows us to break down complex tasks into smaller, more manageable pieces of code. In addition, they provide a way to organize and encapsulate logic in our programs, making them easier to read, write, and maintain.
In this blog, we have covered the basics of methods in Java, including method syntax, return types, and access modifiers. We have also discussed various types of methods, such as static, instance, and constructor methods, along with their individual use cases.
Furthermore, we have explored method overloading, a powerful feature in Java that allows us to define multiple methods with the same name but different parameters based on the arguments' number, order, or data types. This enables us to write more flexible and reusable code, making our programs more robust and efficient.
Understanding the fundamental concepts of methods and their various applications allows us to write more efficient and maintainable code and build more complex and sophisticated programs.
Monitor your Java application for errors & exceptions with Atatus
With Atatus Java performance monitoring, you can monitor the performance and availability of your Java application in real-time and receive alerts when issues arise. This allows you to quickly identify and troubleshoot problems, ensuring that your application is always running smoothly.
One of the key features of Atatus is its ability to monitor the performance of your Java application down to the individual request level. This allows you to see exactly how long each request is taking to process, as well as any errors or exceptions that may have occurred. You can also see a breakdown of the different components of your application, such as the web server, database, and external services, to see how they are affecting performance.
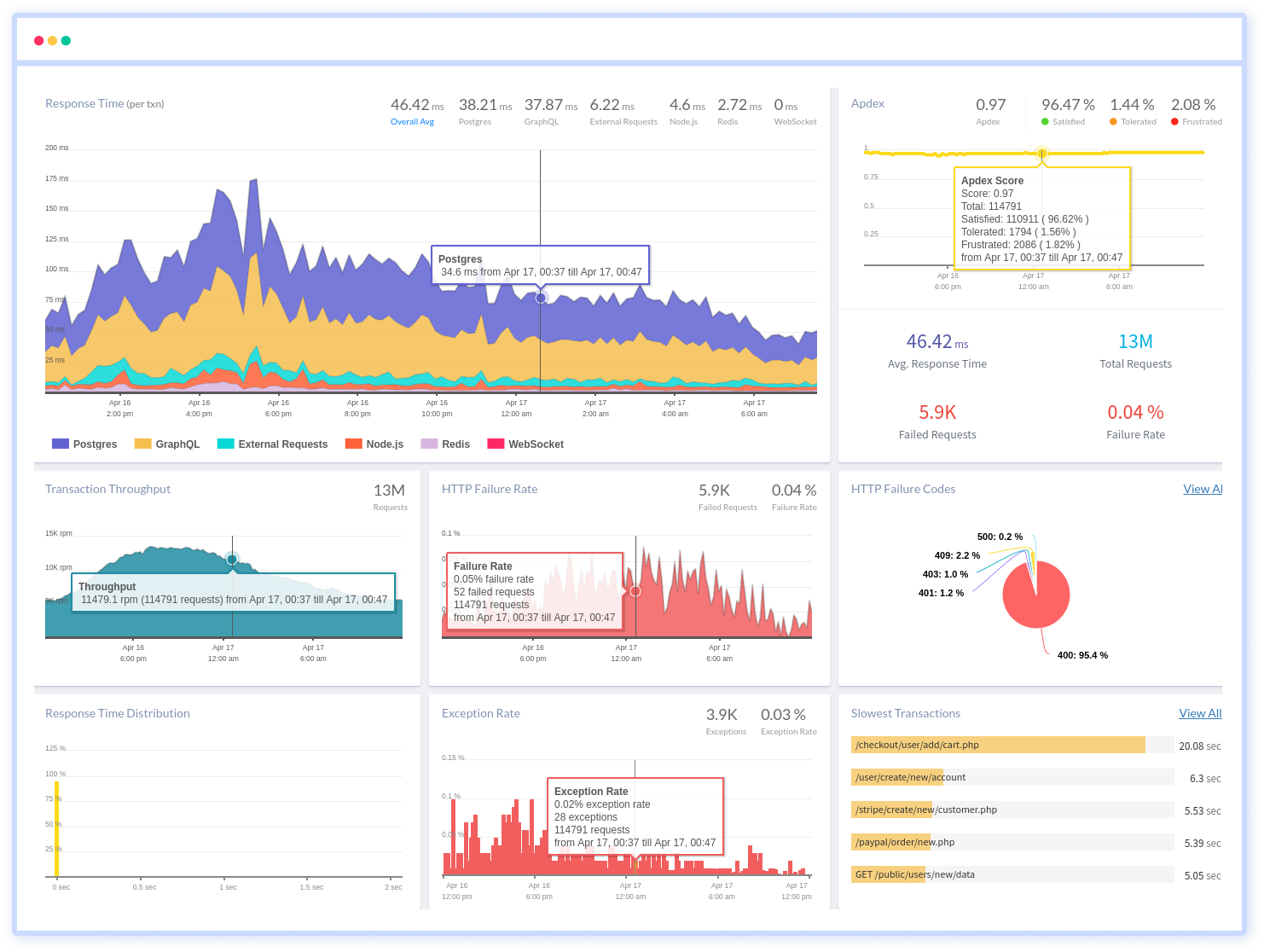
In addition to monitoring performance, Atatus also allows you to track the availability of your Java application. This means that you can see when your application is down or experiencing errors, and receive alerts when this occurs. You can also see how long your application has been running, as well as any uptime or downtime trends over time.
Atatus also offers a range of tools and integrations that can help you to get the most out of your monitoring. For example, you can integrate Atatus with popular tools like Slack, PagerDuty, and Datadog to receive alerts and notifications in your preferred channels. You can also use Atatus's APIs and SDKs to customize your monitoring and build custom integrations with your own tools and systems.
Overall, Atatus is a powerful solution for monitoring and managing the performance and availability of your Java application. By using Atatus, you can ensure that your application is always running smoothly and that any issues are quickly identified and resolved.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More