16 JavaScript Shorthand Coding Techniques
The secret to being a good developer is to write fewer lines of code while accomplishing the task. Thus, shorthand techniques allow you to write a code that is clean and well optimized. To make comparing the javascript shorthand codes and the longhand codes easier, here are some javascript shorthand codes. Learn to use shorthand forms whenever possible.
Table of contents
- Declaring variables and assigning values
- Ternary operator
- Swapping variables
- For loop
- Arrow function
- Embedding variable in string
- Object property
- Spread Operator
- Short circuit evaluation
- String to int conversion
- String repetition
- Multi-line strings
- Switch case alternative
- Exponent power
- Return shorthand
- Shorthand for array.find()
1. Declaring variables and assigning values
The declaration of variable a and b can be done in a single line itself using shorthand.
// variable declaration longhand
let a;
let b = 100;
// shorthand
let a,b = 100;
If Multiple variables need to be assigned the same values, and this can be accomplished using shorthand in one line as well.
// Assigning values to
// multiple variables
// longhand
let a = 100;
let b = 100;
let c = 100;
// shorthand
let a = b = c = 100;
If the variables are of different values then we cannot assign it like we did here. We have to separate the values using commas and still end it writing within a line.
2. Ternary operator
The ternary operator reduces the need to use many lines of code. It actually replaces the if else statement.
// longhand
const age = 20;
let canVote;
if (age > 18) {
canVote = "Eligible for voting";
} else {
canVote = "Not eligible to vote";
}
These many lines can be shrunk into a single line using the ternary operator for shorthand.
// shorthand
const canVote = age > 18 ? "Eligible for voting" : "Not eligible to vote.";
3. Swapping variables
In order to swap two variables, we use another variable and it increases quite a few lines of code. But the same thing can be done with array format in shorthand.
// swap two variables
let a = 10, b = 20;
// longhand
const temp = a;
a = b;
b = temp;
// shorthand
[a, b] = [b, a];
4. For loop
The for loop concept can be simplified using shorthand and written in shortened form.
// for loop
// longhand
const subjects = ['English', 'Math', 'Science'];
for (let i = 0; i < subjects.length; i++) {
console.log(subjects[i]);
}
// shorthand
for (let sub of subjects) {
console.log(sub)
}
If only the index needs to be accessed, then it can be written as follows.
for (let index in subjects) {
console.log(index);
}
5. Arrow function
The arrow function can be used to write our code in shorthand. It is demonstrated as follows.
// longhand
function add(a, b) {
return a + b;
}
// shorthand
const add = (a,b) => a + b;
6. Embedding variable in string
It is easy to embed a variable inside a string using ${ }.
const name = "Sheela";
const age = 21;
const sentence = `${name} is ${age} years old.`;
Output: Sheela is 21 years old.
7. Object property
Objects, being the commonly used data types can be made simpler using shorthand.
// longhand
const name = 'Sheela';
const age = 21;
const gender = 'female';
const myObj = {
Name: name,
Age: age,
Gender: gender
}
// shorthand
const name = 'Sheela';
const age = 21;
const gender = 'female';
const myObj = {name, age, gender}
8. Spread operator
We can merge arrays easily using the spread operator.
let array1 = [10, 20, 30];
// longhand
let array2 = array1.concat([40, 50, 60]);
// shorthand
let array2 = [...array1, 40, 50, 60];
In the longhand method, we have to use concat() in order to merge two arrays. But the spread operator comes to rescue in our shorthand method.
// longhand
Output: [10,20,30,40,50,60]
// shorthand
Output: [10,20,30,40,50,60]
9. Short circuit evaluation
It means evaluating the expressions from left to right using || or && operators.
var x; // undefined
var y = null; // null
var z = undefined; // undefined
var a = 20; // number
var b = 'Hello';
var res = x || y || z || a || b;
console.log(res);
Here, we can see that x is undefined, y is null and z is also undefined. So, the code checks the next line which is var a = 20; and assigns res to the value 20. But it will get short circuited in the same line and wont reach b. So, the output is 20.
10. String to int conversion
If we declare a variable as string, say
var x = "10";
By giving typeof() function, we can find that the variable is stored as a “string”.
In longhand, we can do it as follows:
// longhand
var x = "10";
y = parseInt(x);
console.log(typeof(y));
Output:
number
Same we can simplify it in shorthand like:
// shorthand
var x = "10";
y = +x;
Instead of using parseInt(), this is a shorthand way to convert string into int in javascript.
11. String repetition
To repeat a string, we have to write a certain amount of code using for loop.
// longhand
let strg = ' ';
for(let i = 0; i < 3; i ++) {
strg += 'Hello \n';
}
console.log(strg);
It can be further simplified by using repeat().
// shorthand
let strg = "Hello \n";
a = strg.repeat(3);
console.log(a);
Output:
Hello
Hello
Hello
12. Multi-line strings
In order to write multiple lines of strings in our code, we tend to use the longhand method like this.
// longhand
let name = 'HTML and CSS'
let percentage = 97
const str = 'JavaScript, often abbreviated JS,\n\t'
+ 'is a programming language that is one of the core technologies\n\t'
+ 'of the World Wide Web, alongside ' + name + '. Over ' + percentage + '% of websites\n\t'
+ 'use JavaScript on the client side for web page behavior,\n\t'
+ 'often incorporating third-party libraries.'
But in javascript, we can accomplish this in shorthand using just backticks.
// shorthand
let name = 'HTML and CSS'
let percentage = 97
const str = `JavaScript, often abbreviated JS,
is a programming language that is one of the core technologies
of the World Wide Web, alongside ${name}. Over ${percentage}% of websites
use JavaScript on the client side for web page behavior,
often incorporating third-party libraries.`
13. Switch case alternative
The usual switch case statement looks like this.
// longhand
const dayOfWeek = 3;
switch (dayOfWeek) {
case 1:
document.write("It's Monday");
break;
case 2:
document.write("It's Tuesday");
break;
case 3:
document.write("It's Wednesday");
break;
case 4:
document.write("It's Thursday");
break;
case 5:
document.write("It's Friday");
break;
}
Using shorthand, we can simplify the above switch case statement as follows:
// shorthand
const case = {
'one': () => console.log('It is Monday'),
'two': () => console.log('It is Tuesday'),
'three': () => console.log('It is Wednesday'),
'four': () => console.log('It is Thursday'),
'five': () => console.log('It is Friday')
};
(case[dayOfWeek] || case['three'])();
14. Exponent power
The exponent power function in javascript is Math.pow(). We have to call this every time we want to get the exponent power value.
// longhand
Math.pow(2,5) // 32
In shorthand, we can do it without using the above function.
// shorthand
(2**5) // 32
15. Return shorthand
Return is used to return the result of a function. Let us see the long hand form of this function.
// longhand
function calcCircle(radius) {
return Math.PI * radius * radius
}
To evaluate the multi line code into a single statement, we should use ( ) instead of using { } to wrap the content.
// shorthand
calcCircle = radius=>(Math.PI * radius * radius)
In both cases, calcCircle acts as an user-defined function, and we can call it as follows:
16. Shorthand for array.find()
In the given array, if we have to find a particular object by its name, we have to write a few lines of code to execute it. Let’s see the code of this as follows:
// longhand
const usernames = [
{ firstname: 'Mary', lastname: 'Jones'},
{ firstname: 'John', lastname: 'Smith'},
{ firstname: 'Susan', lastname: 'Brown'},
{ firstname: 'William', lastname: 'Parker'},
]
function findName(name) {
for(let i = 0; i < usernames.length; ++i) {
if(usernames[i].firstname === 'John') {
return usernames[i];
}
}
}
console.log(findName('John'))
The output is for this function is:
Output:
{firstname: 'John', lastname: 'Smith'}
These many lines of code in the findName function can be minimized using shorthand technique as follows:
// shorthand
const usernames = [
{ firstname: 'Mary', lastname: 'Jones'},
{ firstname: 'John', lastname: 'Smith'},
{ firstname: 'Susan', lastname: 'Brown'},
{ firstname: 'William', lastname: 'Parker'},
]
console.log(usernames.find(username => username.firstname === 'John'))
Conclusion
Using shorthand makes our code looks clean and easy to read. But it is not for everyone. If you are a beginner, writing shorthand is not a good idea at first.
Initially you have to learn to code in longhand forms and then once you are strong in it, then you can start using shorthands.
If you want to read more on these kinds of topics, please check this.
Our motive should be to write clean and readable code which is easy to understand by other developers too.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
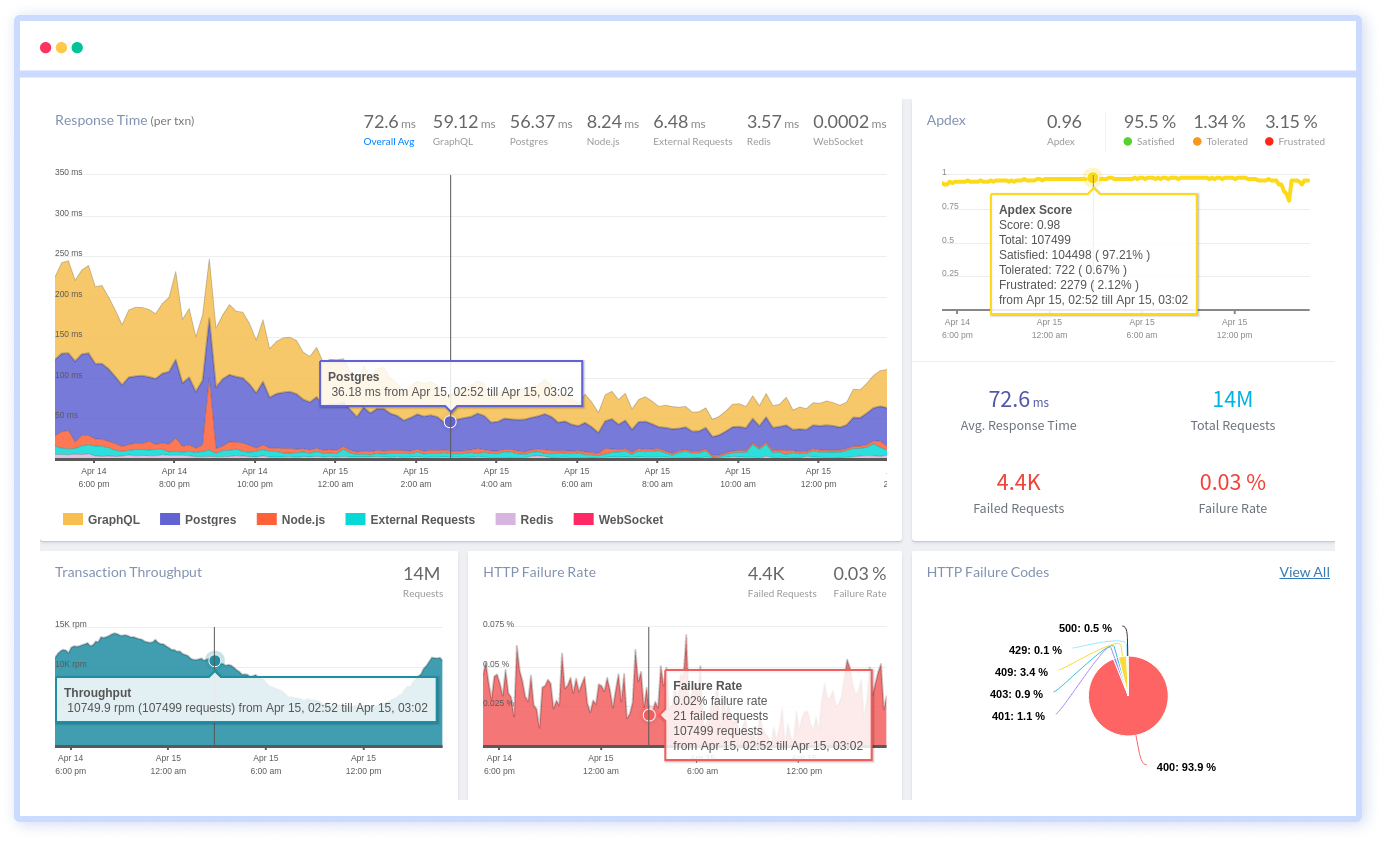
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet a Atatus customer, you can sign up for a 14-day free trial .
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More