PHP is a powerful language used by at least 80% of all the applications you use today as their backend scripting language. This can be attributed to its relatively simpler syntax, which is on the same line as other C-style languages.
It supports different operating systems (Windows, Linux, macOS), web servers, databases and integrates seamlessly with popular databases like MySQL, PostgreSQL, and Oracle, making it a suitable choice for database-driven applications.
PHP scripts can easily be inserted into HTML files, allowing developers to transform static website code into dynamic front-end applications.
Along with this, PHP is open-source. This reduces development costs since there are no licensing fees associated with PHP itself. Additionally, hosting providers offer PHP support by default, making deployment and hosting affordable.
PHP has high market demand, making popular enterprises like Facebook and Wikipedia rely on PHP for their backend data storage.
PHP also has a large and active community of developers worldwide. This vibrant community contributes to the development of frameworks, libraries, and tools that enhance productivity and provide solutions for various use cases.
PHP has been in the market for about 20 years now, and with all these years, there have been some security challenges as well. This blog is dedicated to identifying all those common vulnerabilities and finding suitable solutions to fix them right away.
Table Of Contents
- Cross-Site Scripting (XSS)
- SQL Injection
- Cross-Site Request Forgery (CSRF)
- Remote Code Execution (RCE)
- File Inclusion Vulnerabilities
- Session Hijacking
- Insecure Direct Object References (IDOR)
- Invalidated File Uploads
- Insecure Cryptography
- Code Injection
#1 Cross-Site Scripting (XSS)
Cross-Site Scripting (XSS) is a common PHP security vulnerability that occurs when user-supplied data is not properly sanitized and displayed on a web page without encoding. Attackers can inject malicious scripts, compromising the user's browser and potentially stealing sensitive information or performing unauthorized actions.
How to fix it?
- Properly sanitize user input before displaying it on web pages using functions like
htmlspecialchars()
or a dedicated HTML filtering library. - Implement output encoding based on the context (HTML, JavaScript, CSS) to prevent malicious code execution.
- Use Content Security Policy (CSP) headers to restrict the sources of content that can be loaded.
We will look at an example where users can comment on any topic in a certain website. It does not have any proper sanitation facilities.
<?php
// Vulnerable code
$comment = $_POST["comment"]; // User-supplied comment
echo "Comment: " . $comment; // Display the comment on the webpage
?>
The attacker can use the vulnerable $comment
to inject a malicious Javascript code here.
<script>
// Malicious JavaScript code
alert('XSS Attack!');
// The attacker could also steal sensitive data or perform other malicious actions here
</script>
To prevent such XSS attacks, you can use functions like htmlspecialchars
that prevent running such malicious HTML characters.
<?php
// Corrected code with output encoding
$comment = $_POST['comment']; // User-supplied comment
echo "Comment: " . htmlspecialchars($comment, ENT_QUOTES, 'UTF-8');
?>
#2 SQL Injection
Inadequate validation and sanitization of user-supplied data in PHP can lead to SQL injection, a critical security vulnerability that empowers attackers to execute unauthorized database operations, such as extracting data, modifying records, or even deleting the entire database.
How to fix it?
- To mitigate the PHP vulnerability of SQL injection, it is recommended to use prepared statements or parameterized queries with placeholders. This approach effectively separates the SQL logic from data values, eliminating direct user input in SQL queries and greatly reducing the risk of SQL injection attacks.
- Utilize the PHP Data Objects (PDO) or MySQLi extensions, which support prepared statements and parameter binding.
Here's an example of SQL injection in PHP:
<?php
$query = "SELECT id, name, inserted, size FROM products
WHERE size = '$size'";
$result = odbc_exec($conn, $query);
?>
The static part of the query can be merged with another SELECT statement, which will disclose all passwords:
'
union select '1', concat(uname||'-'||passwd) as name, '1971-01-01', '0' from usertable;
--
UPDATE
and INSERT
statements are also susceptible to such attacks.
#3 Cross-Site Request Forgery (CSRF)
CSRF involves tricking a user's browser into making unintended requests on a trusted website where the victim is authenticated. Attackers exploit the trust relationship to perform unauthorized actions on behalf of the victim without their consent.
How to fix it?
To address the PHP vulnerability of Cross-Site Request Forgery (CSRF), it is important to include a unique CSRF token in all forms and AJAX requests
- Verify the CSRF token on the server-side before processing the request.
- Use techniques like SameSite cookies and anti-CSRF libraries to provide additional protection against CSRF attacks.
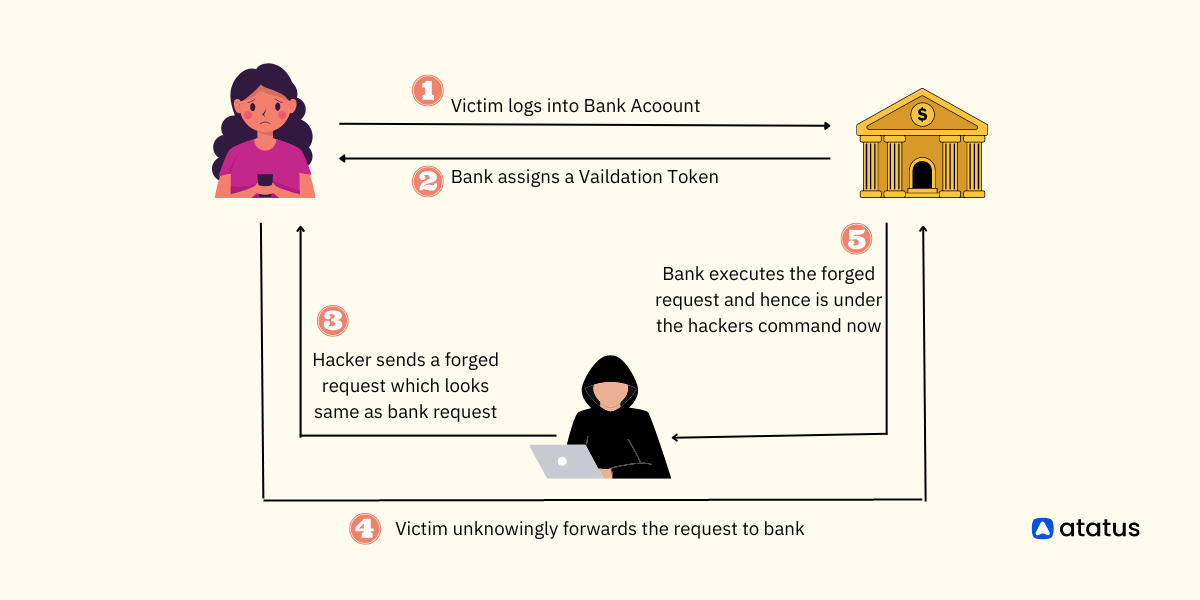
Here's an example of a CSRF vulnerability in PHP:
In this example, let's consider a typical GET request for a $5,000 bank transfer:
GET https://abank.com/transfer.do?account=RandPerson&amount=$5000 HTTP/1.1
An attacker can modify the request to transfer $5,000 to their own account. The malicious request would look like this:
GET https://abank.com/transfer.do?account=SomeAttacker&amount=$5000 HTTP/1.1
The attacker can then embed the request into a seemingly harmless hyperlink:
<a href="https://abank.com/transfer.do?account=SomeAttacker&amount=$5000">Click for more information</a>
The next step for the attacker is to distribute this hyperlink via email to a large number of bank clients. When recipients who are logged into their bank accounts click on the link, they unintentionally initiate the $5,000 transfer.
If the bank's website only uses POST requests, it's not possible to create malicious requests using the <a>
href tag. However, the attack can still be carried out using a <form>
tag.
Here's an example of such a form, which can even be self-submitting:
<body onload="document.forms[0].submit()">
<form id="csrf" action="https://abank.com/transfer.do" method="POST">
<input type="hidden" name="account" value="SomeAttacker"/>
<input type="hidden" name="amount" value="$5000"/>
</form>
</body>
Since the form lacks a submit button, it will be triggered without the user's knowledge and consent. Instead, a single line of JavaScript replaces the button:
document.getElementById('csrf').submit();
#4 Remote Code Execution (RCE)
RCE vulnerabilities pose a significant PHP security risk, as they enable attackers to execute arbitrary code on the server. When user input is not adequately validated or shell commands are executed without proper sanitization, an attacker can exploit these vulnerabilities to execute malicious code, compromising the PHP security of the server.
How to fix it?
- Ensure that user input is properly validated and sanitized before being used in functions or methods that execute commands on the server.
- Avoid executing shell commands directly with user input. If necessary, use safe alternatives like
escapeshellarg()
orescapeshellcmd()
to escape user input.
Here's an example of a remote code execution vulnerability in a hypothetical web application using PHP:
<?php
// This is a vulnerable PHP script
$userInput = $_GET['name'];
$command = "ls -l " . $userInput;
$output = shell_exec($command);
echo "<pre>$output</pre>";
?>
An attacker can exploit this vulnerability by injecting arbitrary shell commands as the name
parameter. For instance, they could manipulate the URL to include a command like http://example.com/vulnerable-script.php?name=;cat+/etc/passwd
.
The resulting command executed by the server would be:
ls -l ;cat /etc/passwd
As a consequence, the server would execute the unintended cat /etc/passwd
command, which displays the contents of the /etc/passwd
file, potentially revealing sensitive information like usernames and hashed passwords.
#5 File Inclusion Vulnerabilities
The dynamic file inclusion functions in PHP can become a PHP vulnerability if user-supplied data is not effectively validated. Attackers can exploit this vulnerability by manipulating the file path, allowing them to include malicious files from external sources.
This opens the door to potential arbitrary code execution or unauthorized access to sensitive files, highlighting the importance of robust PHP security measures.
How to fix it?
- Avoid including files based on user-supplied input. If dynamic file inclusion is required, validate and sanitize the input before using it in file inclusion functions.
- Restrict the inclusion to specific directories to prevent unauthorized access to sensitive files.
- Consider using a whitelist approach to limit the allowed files that can be included.
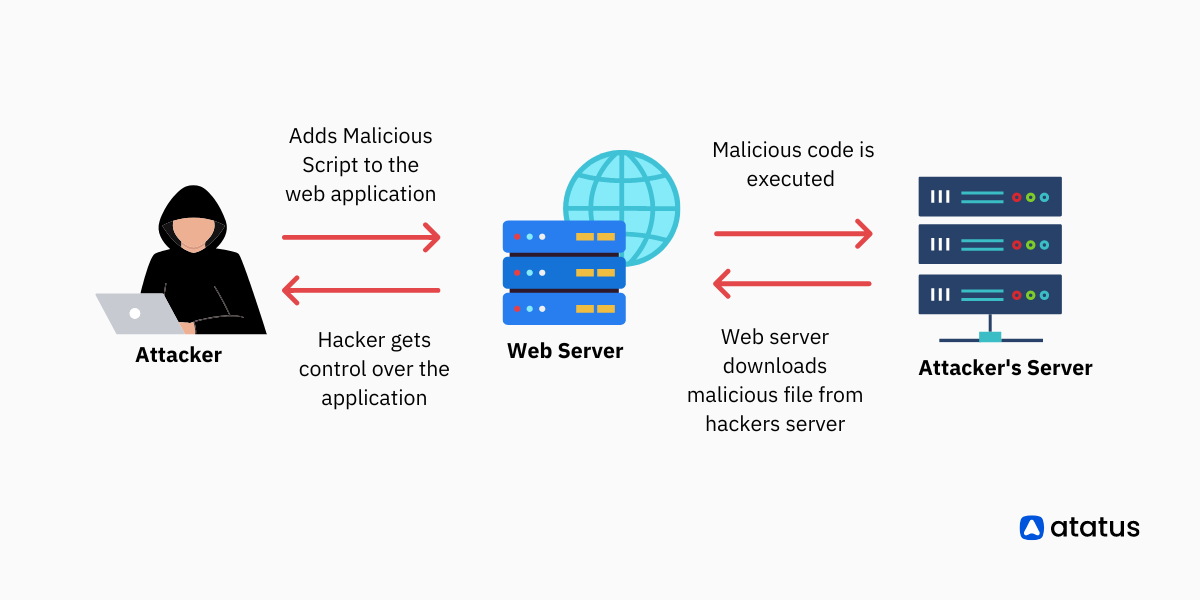
#6 Session Hijacking
Session hijacking, a critical PHP security concern, involves the illicit acquisition of a user's session identifier. Techniques like session fixation or session sidejacking are employed by attackers to obtain the session ID, enabling them to impersonate the user and gain unauthorized access to their account.
How to fix it?
- Regenerate the session ID after a user authenticates, logs in, or changes privilege levels.
- Enhance PHP security by implementing secure session handling techniques such as using secure cookies, enabling HTTP-only flags, and configuring appropriate session options.
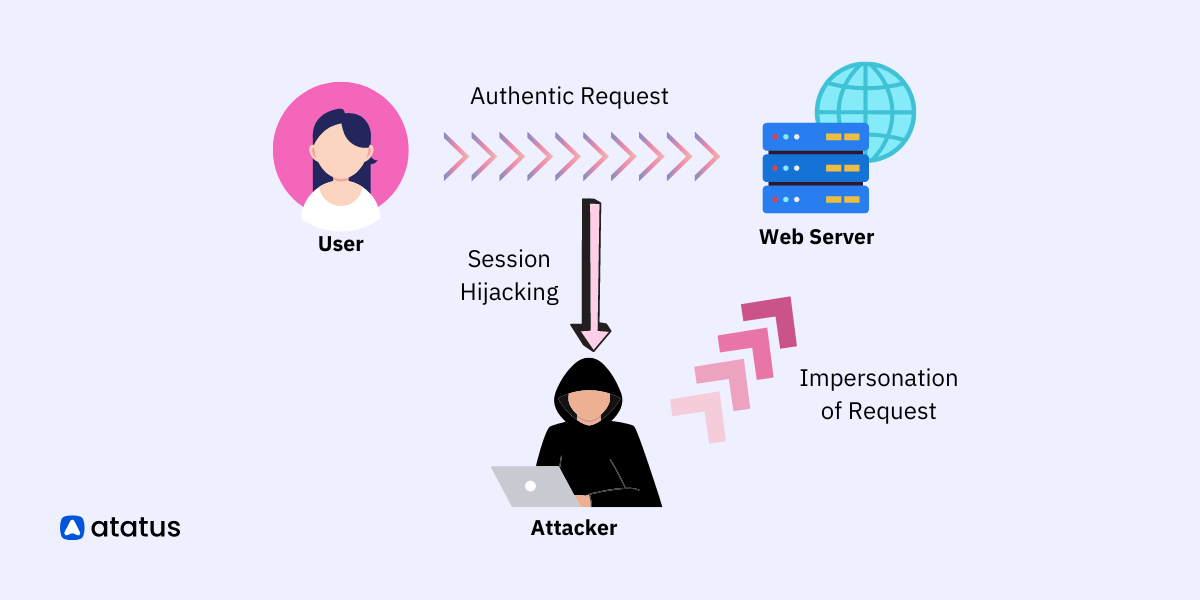
Here's a concise example of session hijacking in PHP:
// Attacker's script
<?php
session_id('attacker_session_id'); // Set the session ID to the attacker's session ID
session_start();
// Perform malicious actions
?>
In this example, the attacker's script sets the session ID to the value of the attacker's session ID using session_id()
. Then, session_start()
is called to start the session with the hijacked session ID. With the session hijacked, the attacker can perform malicious actions, such as accessing the victim's sensitive information or impersonating their identity.
#7 Insecure Direct Object References (IDOR)
An IDOR PHP vulnerability occurs when an application exposes internal object references directly in the URL or parameters. Attackers can manipulate these references to access unauthorized resources or perform actions they should not have permissions for.
How to fix it?
- Avoid exposing internal object references directly in URLs or parameters.
- Implement proper access controls and permissions checks to prevent unauthorized access to sensitive resources.
- Use indirect object references or enforce access control on the server-side.
Here's an example of an Insecure Direct Object Reference (IDOR) vulnerability in PHP:
// Insecure PHP code
<?php
$id = $_GET['id']; // User-supplied input for fetching an object
$query = "SELECT * FROM products WHERE id = $id"; // Insecure direct object reference
$result = mysqli_query($connection, $query);
$product = mysqli_fetch_assoc($result);
// Display product details
?>
In this example, the PHP code takes an id
parameter from the user's input via the GET method. It directly incorporates this input into a SQL query without proper validation or authorization checks. This creates an IDOR vulnerability, as an attacker can manipulate the id
parameter to access unauthorized objects or sensitive data.
To fix this vulnerability, proper authorization and access control should be implemented, ensuring that the user has permission to access the requested object. For example, verifying the user's role or ownership of the object before executing the query would mitigate the IDOR vulnerability.
#8 Invalidated File Uploads
Failure to adequately validate file uploads in PHP can result in a PHP vulnerability. Attackers can take advantage of this vulnerability by disguising malicious files as legitimate ones during the upload process.
Such actions can expose code execution vulnerabilities or enable attackers to overwrite critical files, emphasizing the importance of robust PHP security practices.
How to fix it?
Validate file uploads by checking the file type, size, and content integrity.
- Store uploaded files outside the web root or apply appropriate access controls to prevent direct execution.
- Consider using a secure file upload library or component that handles file validation and security checks.
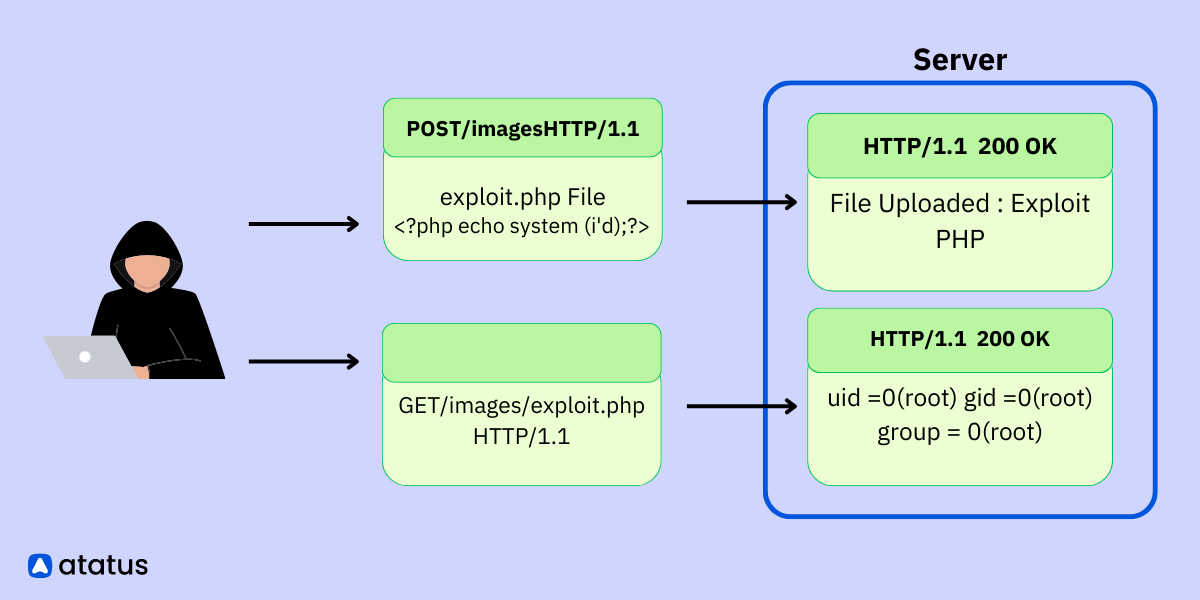
#9 Insecure Cryptography
The use of weak encryption algorithms, poor key management practices, or flawed implementation of cryptographic functions in PHP can expose a PHP vulnerability.
Such vulnerabilities can undermine the security of sensitive data, such as passwords or session tokens, underscoring the importance of employing strong encryption protocols and following best practices for cryptographic operations.
How to fix it?
- Use strong encryption algorithms and secure hashing functions.
- Store passwords and sensitive information as hashed values with a unique salt.
- Implement secure key management practices and ensure the confidentiality and integrity of cryptographic keys.
#10 Code Injection
Similar to SQL injection, code injection occurs when user-supplied data is executed as code without proper validation. Attackers can inject arbitrary PHP code, leading to code execution vulnerabilities or unauthorized access to sensitive information.
How to fix it?
- Avoid executing user input as code.
- Sanitize and validate user input to prevent code injection vulnerabilities.
- Use proper input validation techniques and avoid using eval() or other similar functions.
The example given below demonstrates a web shopping portal on which customers can browse the products of their choice by querying SEARCH. The website will then dropdown a list of similar items and you can select from them.
All good! But the problem is that the $query
is very loosely protected. Because you can search for literally anything on that.
<?php
// Vulnerable code
$searchQuery = $_GET["query"]; // User-supplied search query
$query = "SELECT * FROM products WHERE name LIKE '%" . $searchQuery . "%'";
$result = mysqli_query($connection, $query); // Execute the query
// Display the search results
while ($row = mysqli_fetch_assoc($result)) {
echo $row["name"] . "<br>";
}
?>
The hacker takes advantage of the vulnerable HTTP code and injects the code he wants to:-
http://example.com/search.php?query=anything'; DROP TABLE products; --
But there is always a way out of all this. You can prevent such Code Injection attacks by including more statements and parameterized queries to validate user queries more efficiently.
<?php
// Corrected code with prepared statement
$searchQuery = $_GET['query']; // User-supplied search query
$query = "SELECT * FROM products WHERE name LIKE CONCAT('%', ?, '%')";
$stmt = mysqli_prepare($connection, $query);
mysqli_stmt_bind_param($stmt, 's', $searchQuery);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
// Display the search results
while ($row = mysqli_fetch_assoc($result)) {
echo $row['name'] . '<br>';
}
?>
These are some of the most common security threats and vulnerabilities associated with PHP programs. Fixing these vulnerabilities as soon as you get to know about them will save you from bigger accidents.
However, as the saying goes, “Precaution is always better than cure”. Keeping an eye on your application’s front end and backend regularly will help you stay threat-free for quite a while. With that being said, let me tell you some tips which can be used to improve the security of your application from the get-go.
Improve PHP Security with these tips
Improving PHP security involves implementing various measures to protect your application from common vulnerabilities and threats. Here are some essential steps to enhance PHP security:
- Update your PHP version regularly as a best practice to benefit from security patches addressing known vulnerabilities.
- Review and modify the PHP configuration file (php.ini) to enforce secure settings. Disable dangerous functions, limit resource usage, and enable appropriate error reporting.
- Always validate and sanitize user input to prevent common attacks like SQL injection and cross-site scripting (XSS). Use built-in PHP functions or a dedicated input filtering library to ensure input integrity.
- Instead of concatenating user-supplied data into SQL queries, use prepared statements or parameterized queries with placeholders. This helps prevent SQL injection attacks.
- Implement secure session management techniques, such as generating unique session IDs, setting secure flags for cookies, and regenerating session IDs after user authentication.
- Use output encoding functions, like htmlspecialchars(), when displaying user-generated content to prevent XSS attacks. Consider using Content Security Policy (CSP) headers to restrict the types of content that can be loaded.
- Utilize CSRF tokens in forms and AJAX requests to validate and ensure the authenticity of requests. Implement techniques like SameSite cookies and CSRF protection libraries to mitigate CSRF attacks.
- Enforce proper access controls to limit user privileges and ensure that users can only perform actions they are authorized for. Apply the principle of least privilege, validating user permissions on each request.
- Validate file uploads by checking file types, restricting file sizes, and using secure upload directories. Store uploaded files outside the web root or apply appropriate access controls to prevent direct execution of uploaded files.
- Implement password policies that enforce the use of strong passwords. Hash passwords using secure hashing algorithms (e.g., bcrypt) and consider adding salt for additional security.
- If dynamic file inclusion is necessary, validate and sanitize the input and limit the inclusion to specific directories.
- Perform regular security audits to identify vulnerabilities and conduct penetration testing to assess the robustness of your application's security measures. Address any identified issues promptly.
- Stay updated on the latest PHP security best practices, vulnerabilities, and patches. Follow security blogs, subscribe to security mailing lists, and actively monitor PHP security communities for relevant information.
Utilize Atatus PHP monitoring
Atatus is a SaaS-based application performance monitoring tool. They also offer PHP monitoring and Log management.
Atatus offers an easy-to-install PHP agent that you can integrate into your application. The agent collects performance data and transmits it securely to the Atatus servers for analysis and visualization.
It captures real-time performance data of your PHP application, including response times, throughput, and error rates and provides detailed error logs, stack traces, and contextual information to help you identify and troubleshoot issues quickly.
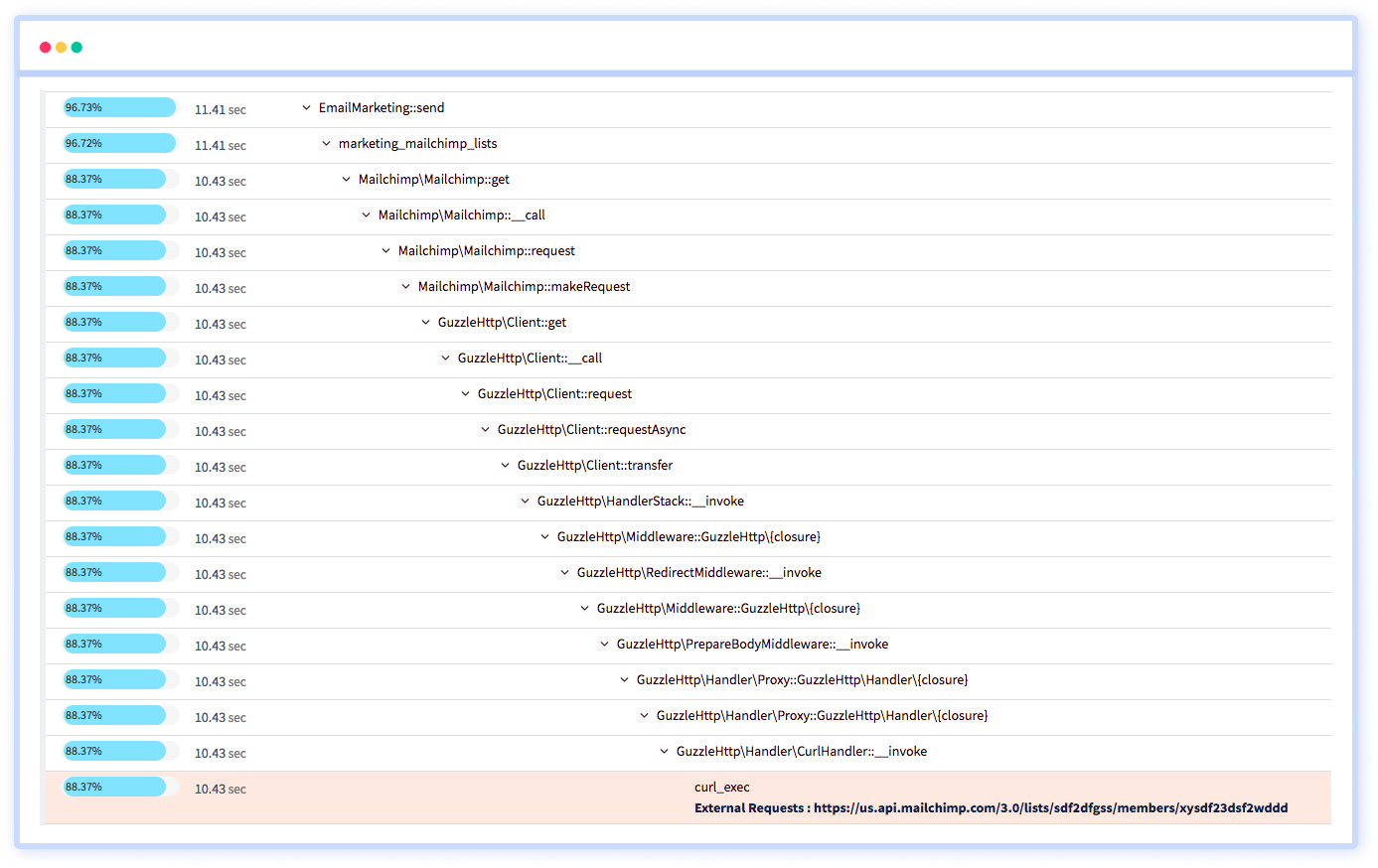
Atatus provides code-level visibility into your PHP application, allowing you to analyze the performance of individual functions, methods, or specific lines of code. This helps you pinpoint performance bottlenecks and optimize critical sections of your application.
Atatus also monitors the performance and availability of external services that your PHP application interacts with, such as APIs or third-party services. It provides insights into the response times and error rates of these services, helping you identify dependencies that may impact your application's performance.
Atatus PHP Monitoring Features:
- Exception Monitoring
- HTTP Failures
- Transaction Monitoring
- Session Traces
- Database Monitoring
- WordPress Hooks
- WordPress Plugins
- Deployment Monitoring
After setting up alerts and notifications, you can receive alerts via various channels like email, Slack, PagerDuty, or custom webhooks, ensuring you stay informed about critical issues.
Conclusion
PHP’s dynamism has certainly created a niche for it among the languages in the programming world. The ability to create cross-platform applications and compatibility with nearly, all the modern servers tops its open-sourceness, making it all the more desirable.
But the looming attackers never miss a chance to cash upon any loophole. The same goes for these application programs. The only thing we can do is to keep a regular check, follow some tested security practices and employ a monitoring tool if needed.
By applying the fixes mentioned in this article and following secure coding practices, you can significantly reduce the risk of vulnerabilities in your PHP application. It's essential to thoroughly test the application after implementing these fixes to ensure that all vulnerabilities have been addressed effectively.
Also, using a monitoring tool like Atatus PHP monitoring will be of great help in the long run. You can gain valuable insights into the performance and stability of your PHP applications, identify and resolve issues promptly, and ensure optimal user experiences.
Remember that securing PHP applications is an ongoing process. You need to maintain a consistent approach to maintaining security.
For other contents on PHP, forage through the topics below:-
- A Complete guide to Debugging PHP
- PHP Profiling: Finding Slow code in your Application
- PHP Exception Handling: Try - Catch and other advanced methods
Atatus: PHP Performance Monitoring and Error Tracking
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance.
Atatus PHP monitoring, tracks and provides real-time notifications for every error, exception, and performance problem that occurs within the application. .
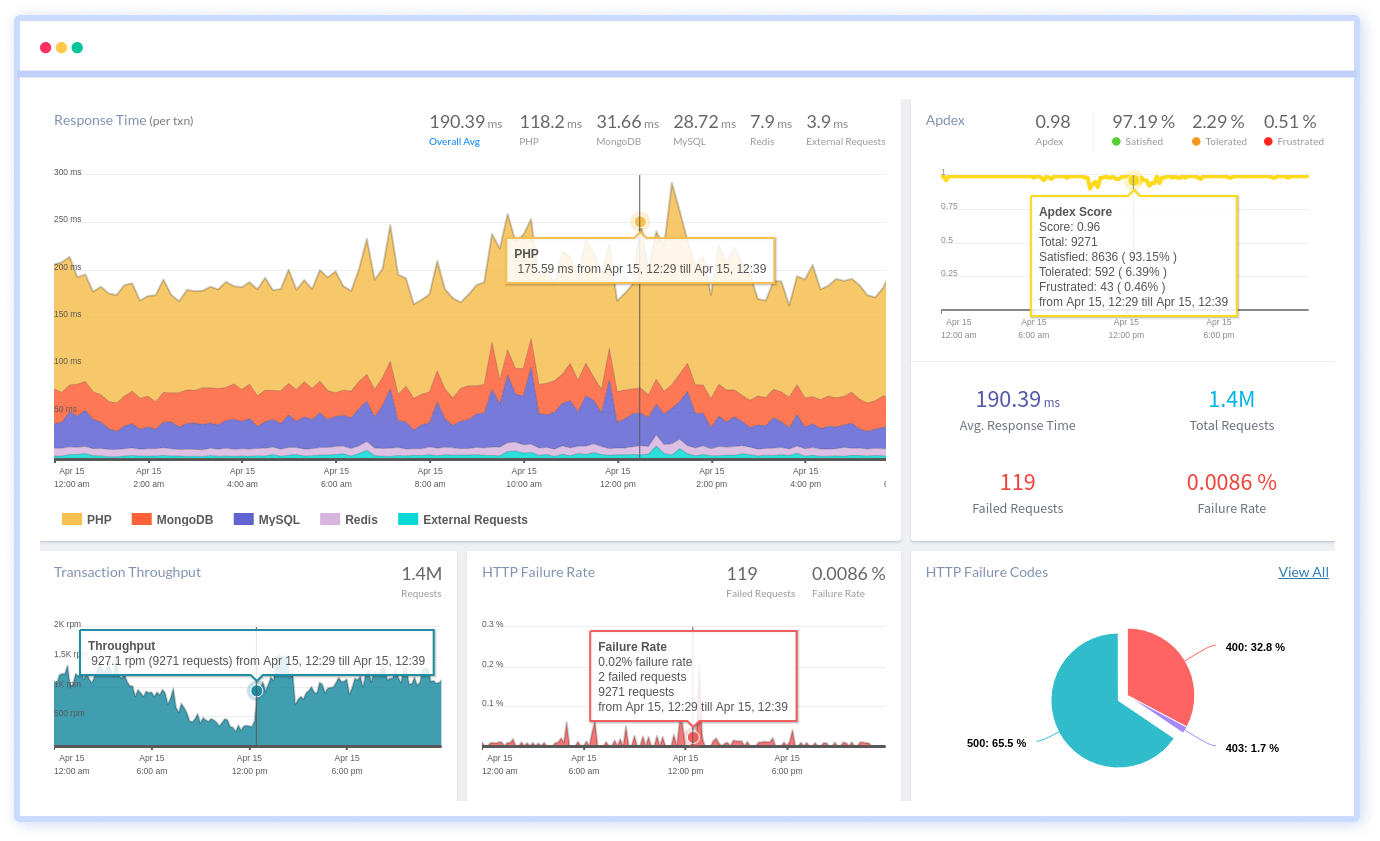
By tracking KPIs such as response times, throughput, HTTP failure rates, and error rates, it can help users identify and address any performance issues before they impact the application's users.
Furthermore, Atatus supports multiple PHP framework such as WordPress, Magento, Laravel, CodeIgniter, CakePHP, Symfony, Yii and Slim making it an adaptable tool that can be used to monitor applications across different frameworks and platforms.