RabbitMQ: The Ultimate Guide to Reliable Message Delivery
Have you ever wondered how modern applications, especially those built on microservices architecture, communicate with each other? In today's fast-paced, distributed computing environment, where services need to be highly available, scalable, and fault-tolerant, traditional communication mechanisms like REST APIs and SOAP may not suffice. This is where message-based communication comes into play.
Message-based communication is a popular approach in microservices architecture, where services communicate with each other by sending and receiving messages asynchronously. This approach provides several benefits, including loose coupling between services, better fault tolerance, and improved scalability.
However, implementing message-based communication can be challenging, especially when dealing with large volumes of messages and multiple services.
This is where RabbitMQ comes in. RabbitMQ is a powerful and flexible message broker that provides reliable and scalable messaging capabilities for microservices architecture.
In this blog, we will explore RabbitMQ in-depth and learn how to use it to implement message-based communication between microservices.
Table of Content
- Messaging Systems and Message Brokers
- RabbitMQ and its Features
- Queues and Exchanges in RabbitMQ
- Understanding Message Routing in RabbitMQ
- Framework Compatibility and Integration
- Comparison of RabbitMQ with Other Message Brokers
Messaging Systems and Message Brokers
Messaging systems and message brokers are essential components of modern distributed systems. They provide a way for different parts of an application to communicate with each other, even if they are running on different machines or in different locations.
Messaging systems work by sending messages between different parts of the application, rather than passing data directly. The message broker acts as a mediator between the components, receiving messages from producers and delivering them to consumers.
Message brokers like RabbitMQ, Apache Kafka and Apache ActiveMQ are some of the most popular messaging systems in use today. They offer a wide range of features that make it easy to implement reliable messaging in complex distributed systems. These features can include:
- Message routing: allowing messages to be delivered to the correct destination based on rules or criteria defined by the application
- Message filtering: allowing messages to be selectively consumed based on their content or metadata
- Message persistence: ensuring that messages are not lost even if the broker or one of its components fails
- Message acknowledgement: providing confirmation to producers that their messages have been successfully delivered
- Message transformation: allowing messages to be translated or converted between different formats or protocols
- Message batching: allowing multiple messages to be sent together as a single unit, reducing the overhead of network communication
In addition to these features, messaging systems can also provide monitoring and management tools to help developers and administrators understand how the system is performing and identify issues before they become critical.
RabbitMQ and its Features
RabbitMQ is a popular open-source message broker software that facilitates communication between applications and services using message queues. It is built on top of the Advanced Message Queuing Protocol (AMQP), a standardized protocol for message-oriented middleware, and is implemented in the Erlang programming language.
RabbitMQ allows applications to send and receive messages through a messaging queue, which serves as a buffer between the sender and the receiver. The messaging queue holds the messages until the receiver is ready to process them, ensuring reliable delivery and reducing the likelihood of data loss.
RabbitMQ provides a wide range of features, including routing, persistence, clustering, message acknowledgement, priority queues, and plugins. These features make RabbitMQ a powerful and flexible messaging system that is ideal for building distributed systems and microservices architectures.
- Routing: RabbitMQ allows messages to be sent to specific queues based on routing keys, which can be used to filter and route messages to the appropriate recipient.
- Persistence: RabbitMQ can store messages on disk, ensuring that they are not lost if the server or network fails.
- Clustering: RabbitMQ can be set up in a cluster, which allows multiple nodes to work together to provide high availability and scalability.
- Message acknowledgement: RabbitMQ supports message acknowledgement, which means that a sender can be notified when a message has been successfully received by the recipient.
- Priority queues: RabbitMQ allows messages to be prioritized, which ensures that high-priority messages are processed before lower-priority messages.
- Plugins: RabbitMQ has a plugin architecture that allows developers to extend its functionality with additional features.
RabbitMQ can be easily integrated with various programming languages, platforms, and frameworks, including Java, .NET, Python, Ruby, Node.js, and more. It also provides a web-based management interface for monitoring and managing the message broker.
Queues and Exchanges in RabbitMQ
Queues and exchanges are fundamental components of RabbitMQ that enable communication between producers and consumers. Queues serve as buffers that hold messages until they are consumed by a consumer, while exchanges route messages to the appropriate queue(s) based on certain criteria.
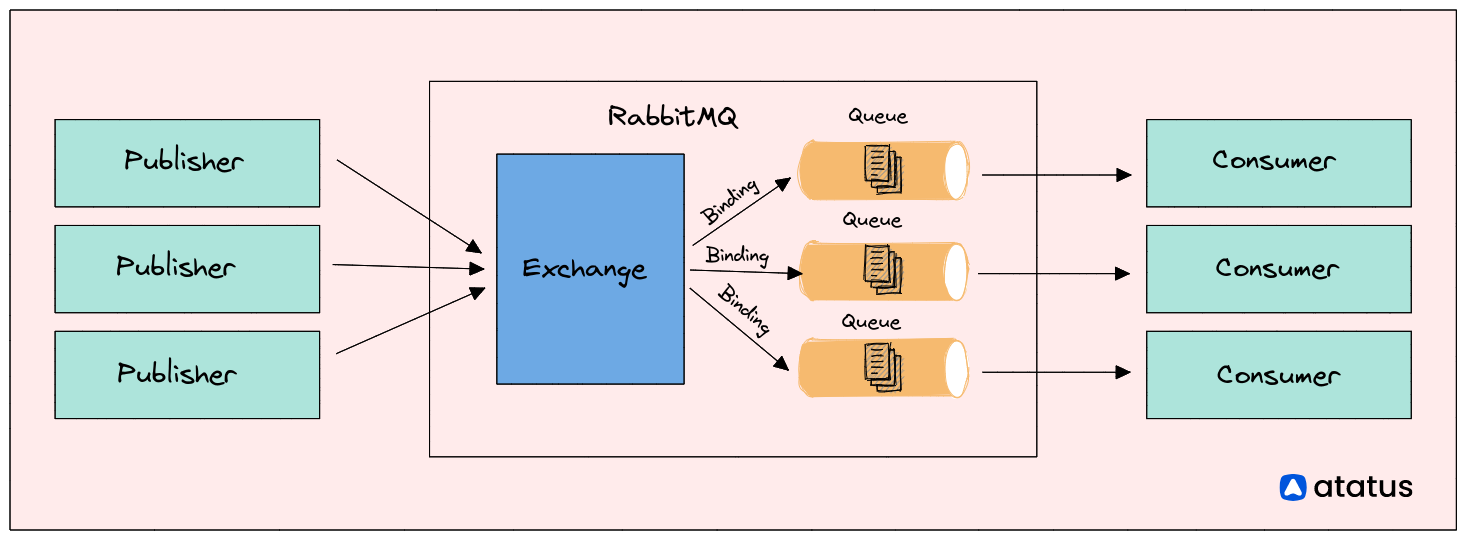
1. Queues
A queue is a data structure that holds messages until they are consumed by a consumer. When a producer sends a message to RabbitMQ, the message is placed in a queue.
The queue then waits for a consumer to consume the message. Queues can be created with a range of configuration options, such as maximum length, maximum message size, and message TTL. Queues can also be durable, which means that they survive a RabbitMQ broker restart.
2. Exchanges
An exchange is a component that receives messages from producers and routes them to one or more queues. Exchanges use a routing key to determine how messages are routed to queues.
Exchanges can be created with different configuration options, such as exchange type, exchange name, and exchange durability. Exchanges can also be bound to queues using a binding key, which specifies how messages should be routed from the exchange to the queue.
Understanding Message Routing in RabbitMQ
In RabbitMQ, message routing refers to the process of delivering messages from a sender to one or more receivers based on certain criteria, such as routing keys or message attributes.
When a message is sent to RabbitMQ, it is published to an exchange. The exchange receives messages from producers and routes them to one or more queues based on the routing rules that are defined for the exchange. These routing rules are specified by the exchange type, which determines how messages are distributed to the queues.
There are four types of exchanges in RabbitMQ:
1. Direct exchange
Direct exchanges in RabbitMQ are designed for one-to-one message routing.
When a message is sent to a direct exchange, it is given a specific routing key. The exchange then delivers the message to the queue that is bound to the exchange with the same routing key. If there are multiple queues bound to the exchange with the same routing key, the message will be delivered to all of them.
Direct exchanges are particularly useful when you need to send messages directly to a specific queue based on certain criteria, such as message content or origin. For instance, you could use a direct exchange in a system that processes different types of messages by different queues, allowing you to route each message type to its respective queue using a predefined routing key.
To create a direct exchange in RabbitMQ, you can specify the routing key to use when binding a queue to the exchange. Additionally, you can create multiple bindings with different routing keys for the same queue, giving you greater control over message routing.
Direct exchanges offer a straightforward and efficient means of routing messages to specific queues in RabbitMQ.
2. Fanout exchange
Fanout exchanges are designed for one-to-many message routing.
In a Fanout exchange, messages are sent to the exchange without any routing key. The exchange then delivers the message to all the queues that are bound to the exchange. Fanout exchanges ignore any routing key specified by the message and instead broadcast the message to all bound queues.
Fanout exchanges are useful in scenarios where you want to broadcast a message to multiple consumers, regardless of any criteria or message content. For example, you could use a Fanout exchange to send a message to multiple instances of a microservice to ensure redundancy or to broadcast a notification to multiple clients.
When creating a Fanout exchange in RabbitMQ, you do not need to specify any routing key. Instead, you simply bind the exchange to the relevant queues that will consume the message. Any message that is published to the exchange will then be sent to all bound queues.
Fanout exchanges provide a simple and efficient way to broadcast messages to multiple queues in RabbitMQ.
3. Topic exchange
Topic exchange in RabbitMQ, is designed to handle complex message routing based on patterns that match the message's routing key. In a Topic exchange, messages are sent to the exchange with a routing key that consists of one or more words separated by dots.
The exchange delivers the message to the queue that is bound to the exchange with a routing key matching the message routing key. The routing key can contain special characters such as *
and #
, enabling flexible pattern matching.
Topic exchange is useful in scenarios where you need to route messages to specific queues based on multiple criteria such as message content, origin, or type. For instance, you can use a Topic exchange to route messages to different queues based on the error's severity level or message type and geographic region combination.
When creating a Topic exchange in RabbitMQ, you can specify the routing key pattern used to bind a queue to the exchange. Additionally, you can create multiple bindings with various routing key patterns for the same queue, providing more control over message routing.
Topic exchanges provide a robust way to route messages based on intricate patterns, making them a preferred choice for advanced message routing scenarios.
4. Headers exchange
Headers exchange is one of the types of message exchanges available in RabbitMQ, which facilitates message routing based on headers, rather than the routing key.
Messages sent to this exchange include headers that have key-value pairs. The exchange delivers these messages to the queue that is bound to the exchange with headers that match the message headers based on Boolean logic provided in the binding.
Headers exchanges can be useful when you need to route messages based on specific header values, such as content type or encoding. However, it's considered less efficient than other exchange types, especially when handling high-traffic scenarios.
While creating a Headers exchange, you can specify the headers to use when binding a queue to the exchange. The exchange delivers the message to the queue if the headers in the message match the headers in the binding based on the Boolean logic provided.
While Headers exchanges provide a way to route messages based on headers, it's not the most efficient method for message routing, and other exchange types such as Direct or Topic exchanges are typically more efficient in most scenarios.
Here's an example of how to use all the different types of exchanges in RabbitMQ using Node.js:
const amqp = require('amqplib');
async function main() {
// Connect to RabbitMQ
const connection = await amqp.connect('amqp://localhost');
const channel = await connection.createChannel();
// Create queues
await channel.assertQueue('queue1');
await channel.assertQueue('queue2');
// Create bindings
await channel.bindQueue('queue1', 'direct-exchange', 'direct-key');
await channel.bindQueue('queue2', 'topic-exchange', 'topic.*');
// Send messages to the exchanges
channel.publish('direct-exchange', 'direct-key', Buffer.from('Hello from direct exchange!'));
channel.publish('fanout-exchange', '', Buffer.from('Hello from fanout exchange!'));
channel.publish('topic-exchange', 'topic.key', Buffer.from('Hello from topic exchange!'));
channel.publish('headers-exchange', '', Buffer.from('Hello from headers exchange!'), { headers: { foo: 'bar' } });
// Consume messages from the queues
channel.consume('queue1', (msg) => {
console.log(`Received message from queue1: ${msg.content.toString()}`);
channel.ack(msg);
});
channel.consume('queue2', (msg) => {
console.log(`Received message from queue2: ${msg.content.toString()}`);
channel.ack(msg);
}, { noAck: false }); // Set noAck to false to require explicit acknowledgement
// Close the connection
setTimeout(() => {
connection.close();
process.exit(0);
}, 5000);
}
main().catch(console.error);
In this example, we create a connection to a local RabbitMQ instance and create two queues, queue1
and queue2
. We then create bindings for these queues on different types of exchanges: a direct exchange, a fanout exchange, a topic exchange, and a headers exchange.
We then send messages to each of these exchanges with different routing keys, and consume the messages from the corresponding queues. Note that for queue2
, we set noAck
to false
to require explicit acknowledgement of messages.
Finally, we close the connection after a short delay.
Discover the importance of RabbitMQ monitoring and get actionable insights to improve your messaging infrastructure.
Framework Compatibility and Integration
RabbitMQ provides support for many programming languages and frameworks through AMQP client libraries and plugins. These client libraries and plugins allow developers to interact with RabbitMQ using their preferred programming language or framework.
For example, if you are building a Java application, you can use the RabbitMQ Java client library to interact with RabbitMQ. Similarly, if you are building a Python application, you can use the Pika library to interact with RabbitMQ.
These libraries and plugins provide a range of features and functionality, such as message publishing, message consumption, and advanced queue and exchange configuration. They also provide support for connection management, error handling, and other features that are critical for building robust and reliable messaging-based applications.
Here are some of the programming languages and frameworks that have RabbitMQ integrations,
- Java: RabbitMQ provides an AMQP client library for Java called the
RabbitMQ Java client
. This client supports a range of features, including message publishing, message consumption, and advanced queue and exchange configuration. - Python: RabbitMQ provides an AMQP client library for Python called
Pika
. This library provides a simple and easy-to-use interface for interacting with RabbitMQ, and supports features such as message publishing, message consumption, and connection management. - Ruby: RabbitMQ provides an AMQP client library for Ruby called
Bunny
. This library provides a simple and efficient way to interact with RabbitMQ, and supports features such as message publishing, message consumption, and error handling. - Node.js: RabbitMQ provides a Node.js AMQP client library called
amqplib
. This library provides a straightforward way to interact with RabbitMQ, and supports features such as message publishing, message consumption, and connection management. - .NET: RabbitMQ provides a .NET AMQP client library called the
RabbitMQ .NET
client. This library provides a powerful and flexible way to interact with RabbitMQ, and supports features such as message publishing, message consumption, and advanced queue and exchange configuration.
Comparison of RabbitMQ with Other Message Brokers
Message-based communication is a popular approach in microservices architecture, where services communicate with each other by sending and receiving messages asynchronously. This approach provides several benefits, including loose coupling between services, better fault tolerance, and improved scalability.
RabbitMQ is an excellent choice for implementing message-based communication in microservices. It provides reliable and scalable messaging capabilities, support for multiple messaging protocols, and flexible routing capabilities.
Here is a comparison of RabbitMQ with some other popular message brokers:
1. Apache Kafka
Apache Kafka is a distributed streaming platform that is designed to handle large volumes of data streams in real-time. Kafka is optimized for write-intensive workloads and is commonly used for event-driven architectures, log aggregation, and real-time analytics.
Compared to RabbitMQ, Kafka provides better support for handling large data volumes and real-time processing. However, Kafka does not provide built-in support for message acknowledgments, which can make it more challenging to ensure message reliability.
2. ActiveMQ
Apache ActiveMQ is an open-source message broker that provides support for multiple messaging protocols, including JMS, AMQP, and MQTT. ActiveMQ is known for its high performance and flexible configuration options.
Compared to RabbitMQ, ActiveMQ provides better support for integrating with Java applications, as it is built on the Java Message Service (JMS) specification. However, RabbitMQ provides better support for complex routing scenarios and is generally considered more flexible and extensible.
3. Apache Pulsar
Apache Pulsar is a distributed messaging platform that is designed for streaming and real-time data processing. Pulsar provides support for multiple messaging protocols and features like message deduplication, geo-replication, and automatic partitioning.
Compared to RabbitMQ, Pulsar provides better support for handling large data volumes and scaling horizontally. However, RabbitMQ provides better support for complex routing scenarios and is generally easier to set up and configure
RabbitMQ remains a popular choice for enterprise applications that require flexible messaging capabilities and high reliability.
Conclusion
We have covered various aspects of using RabbitMQ for message-based communication in a microservices architecture. We started by introducing RabbitMQ and its key features, including support for multiple messaging protocols, queueing, routing, high availability, scalability, and security.
Next, we discussed how to set up RabbitMQ and create queues and exchanges. We also discussed how to integrate RabbitMQ with popular programming languages and frameworks, such as Java, Python, and Node.js. We covered how to scale and monitor RabbitMQ clusters, as well as how RabbitMQ compares to other message brokers.
RabbitMQ is a powerful and flexible message broker that provides reliable and scalable messaging capabilities for microservices architecture. It support for multiple messaging protocols, flexible routing capabilities, and high availability make it a popular choice for implementing message-based communication in modern applications.
Monitor Your Entire Application with Atatus
Atatus is a Full Stack Observability Platform that lets you review problems as if they happened in your application. Instead of guessing why errors happen or asking users for screenshots and log dumps, Atatus lets you replay the session to quickly understand what went wrong.
We offer Application Performance Monitoring, Real User Monitoring, Server Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring, and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
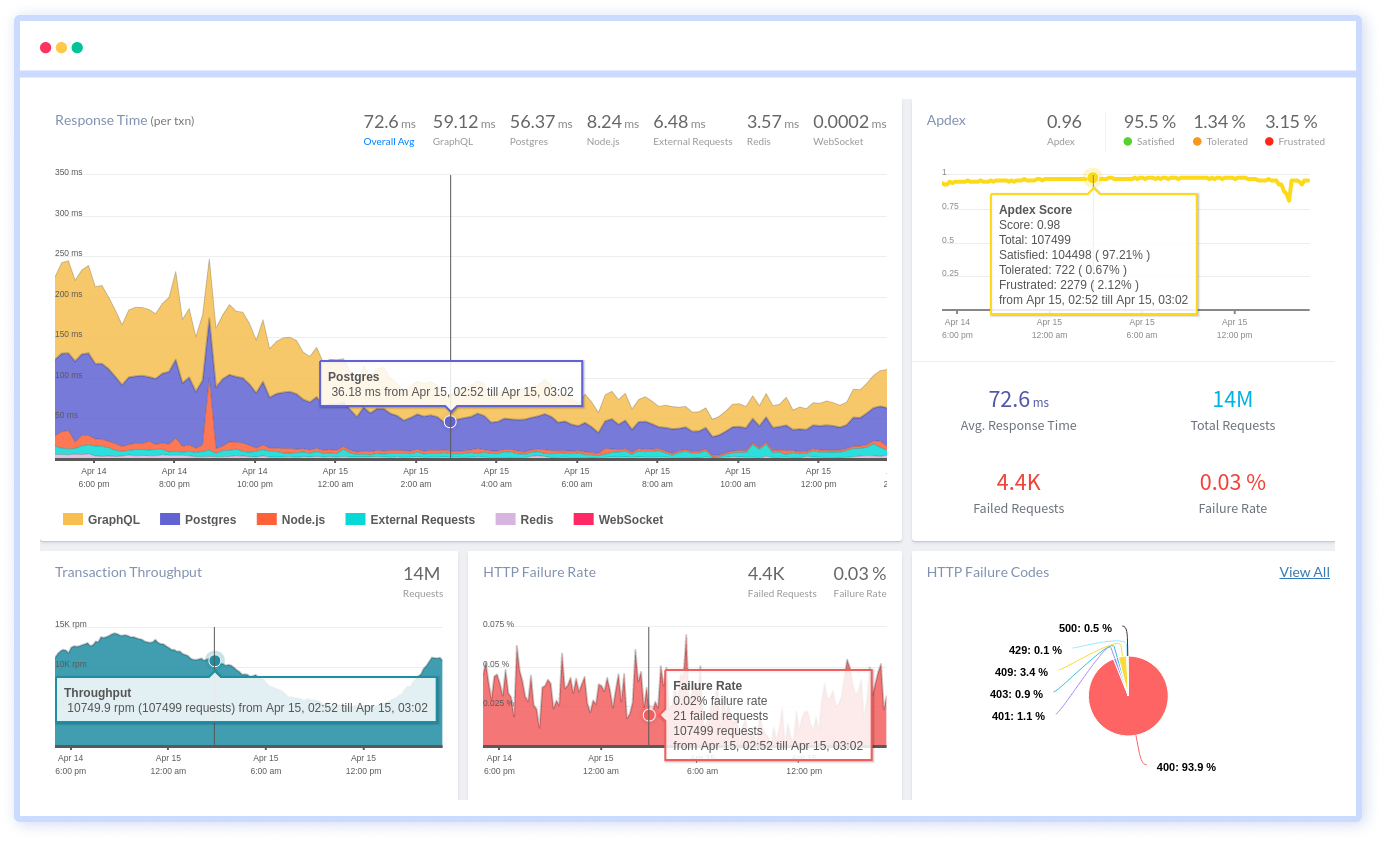
Atatus can be beneficial to your business, which provides a comprehensive view of your application, including how it works, where performance bottlenecks exist, which users are most impacted, and which errors break your code for your frontend, backend, and infrastructure.
If you are not yet an Atatus customer, you can sign up for a 14-day free trial.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More