REST API Best Practices for Parameter and Query String Usage
Over the last ten years, APIs have grown in popularity and utilization. They've evolved into critical components of application infrastructure, and as businesses continue to use and build them, good API architecture will become increasingly important. A smart API design helps performance and the overall developer experience, whether they're public or internal.
The most common APIs employ HTTP requests to access and use data and follow a RESTful architecture. As a result, they're built such that parameters can be used to change HTTP requests to return the desired data, commonly in JSON (JavaScript Object Notation) format.
We will cover the following:
- Introduction
- Parameters
- What Type of Parameter Should We Add?
- How to use Query String Parameters?
- Array and Map Parameters
- When Should the Query String not be Used?
Introduction
Representational State Transfer (REST) is one of the most widely used protocols for building API contracts.
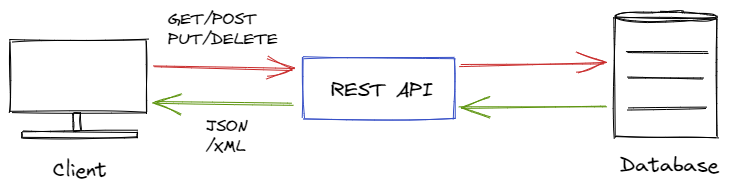
REST differs from competitors like SOAP and RPC in that it is based on the concept of state and relies on the underlying protocol HTTP for other features like action and context. The data that is retrieved via the API might be considered by the state.
Let's look at an illustration of the above concept: an API provides the capability to modify the user's profile picture. The state, or in this case, the data or request payload, would be represented by the user's identification and the new picture.
The action - update and existing state - is represented by the fact that we are updating the picture. The request context includes the client’s authorization for this action.
When building RESTful APIs, it's important to remember that the REST protocol is state-based and strongly reliant on HTTP.
Parameters
Parameters are options you can send to the endpoint to modify the response (for example, selecting the response format or the amount returned).
Different types of parameters are frequently documented in separate groups on the same page. Each type of parameter is not present at every endpoint.
The first thing to do when utilizing an API is to read the documentation. This can help you avoid bugs in the future, and knowing how to use built-in parameters will make your life easier, especially if you're working with a huge database.
Types of Parameters
Parameters can be divided into header parameters, path parameters, and query string parameters.
- Header Parameters
Parameters in the request header that are typically connected to authorization. - Path Parameters
Curly braces are commonly used to separate these. - Query String Parameters
After the question mark (?) parameters in the endpoint's query string.
Although request bodies resemble parameters, they are not officially a parameter.
1. Header Parameters
The request header contains header parameters. The header often only contains authorization parameters that are shared by all endpoints; as a result, the header parameters are rarely documented with each endpoint.
Instead, the authorization needs section documents the authorization details in header parameters. If your endpoint requires specific parameters to be given in the header, you should document them in the endpoint's parameters documentation.
2. Path Parameters
Path parameters are not optional and are part of the endpoint itself. For example, in the following endpoint, the path parameters {user} and {motorcycleId} are required:
/service/myresource/user/{user}/motorcycles/{motorcycleId}
Curly brackets are typically used to separate path arguments, while some API doc styles use a colon or a different syntax. Indicate the default values, allowed values, and other details when documenting path parameters.
Color Coding The Path Parameters
When listing the path parameters in your endpoint, color coding the parameters can make them easier to identify. The color-coding of the parameters makes it apparent what is and isn't a path parameter. You can make an immediate connection between the endpoint and the parameter definitions by using color.
You could, for example, color-code your arguments as follows:
/service/myresource/user/{user}/motorcycles/{motorcycleId}
It's easy to identify which parameter is being specified and how it relates to the endpoint description because the parameters are color-coded.
3. Query String Parameters
After a question mark (?) in the endpoint, query string parameters appear. The "query string" is defined as a question mark followed by the parameters and their values. Each parameter is listed one after the other in the query string, separated by an ampersand (&). It makes no difference what order the query string parameters are in.
For example:
/surfreport/{beachId}?day=sunday&location=florida&time=1400
and
/surfreport/{beachId}?time=1400&location=florida&day=sunday
It would produce the same outcome.
The order of path parameters is important. If the parameter is part of the endpoint itself (rather than being added after the query string), the value is usually specified in the endpoint's description.
What Type of Parameter Should We Add?
The first thing we should consider is the type of parameter we wish to provide.
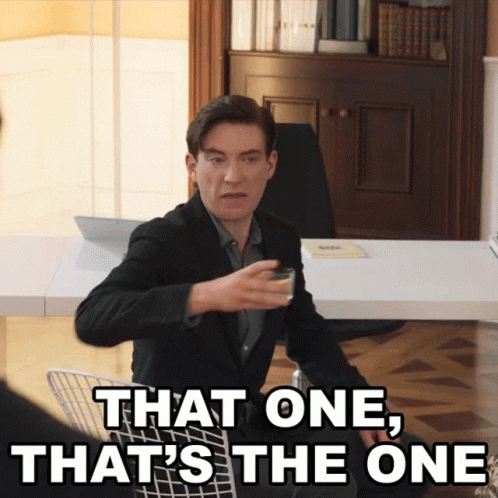
Perhaps it's a parameter that's already established in the HTTP specification as a header field.
There are a lot of standardized fields. We can sometimes re-invent the wheel by moving information to a different location. I'm not saying we can't try something new.
For example, GraphQL does things that we would consider weird from a REST standpoint, but it still works. It's sometimes just easier to use what's already available.
Take the Accept header, for example. This enables us to specify the format, or media type, in which the response should be sent. This can be used to inform the API that JSON or XML is required. We can also use this to get the API's version.
Instead of utilizing a query string as a cache buster (?cb=), we could use the Cache-Control header to prevent the API from providing us a cached answer with no-cache.
Authorization could also be considered a parameter. Depending on the specifics of the API's authorization, allowed or unauthorized answers may change. For this purpose, HTTP defines an Authorization header.
After we've gone over all of the default header fields, we'll need to decide whether we should construct a custom header field for our parameter or put it in the URL's query string.
How to use Query String Parameters?
Always reference the API documentation before utilizing query string parameters. Not all APIs are the same, and not all query string formats are compatible with the API. However, there are a few basic guidelines to follow to get started and make data filtering a breeze.
After the base URL and path parameters, a question mark(?) is added to the endpoint to add query string parameters (if any). The query strings that follow the question mark(?) specify specific parameters and values.
http://api.mycompany.com/{path_parameter}?query_parameter=value
The parameters can be chained on, one after the other, separated by an ampersand(&). It doesn't matter which order the parameters are in.
Query strings differ between APIs. So, once again, it's critical to reference the documentation to see what capabilities are available. Limit, offset, and page are frequent query string parameters in bigger API databases.
Limit specifies how many resources/instances you want to be returned, while offset specifies where the count should begin.
Array and Map Parameters
What to do with array parameters in the query string is a frequently asked question.
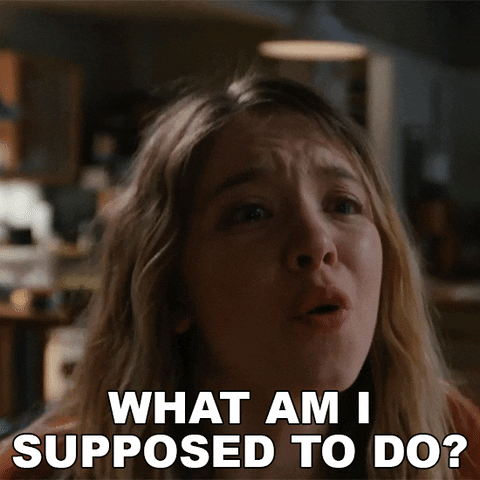
For instance, suppose we want to search for many names.
The usage of square brackets is one option.
/authors?name[]=colleen&name[]=hoover
This fact is overlooked by many HTTP server and client implementations, but it should be remembered.
Another option is to simply use the same parameter name multiple times:
/authors?name=colleen&name=hoover
This is a viable method, however, it may reduce developer experience.
Clients frequently employ a map-like data structure that undergoes a simple string conversion before being appended to the URL, potentially causing the following values to be overridden. Before the request may be issued, a more complicated conversion is required.
Another option is to use, characters to separate the values, which are allowed unencoded inside URLs.
/authors?name=colleen,hoover
The . (dot) character, which is also allowed unencoded, can be used for map-like data structures.
/articles?age.gt=31&age.lt=50
It's also possible to URL-encode the entire query string, allowing us to use any characters or formatting we want. It should be noted that this can significantly reduce developer experience.
When Should the Query String not be Used?
We shouldn't put sensitive data like passwords in the query string because it's part of our URL and can be read by anyone sitting between the customers and the API.
Also, if we don't take URL design and length carefully, the developer experience suffers considerably. Sure, most HTTP clients will let you have a URL with a five-figure length of characters, but debugging such strings is a pain.
Since anything can be declared as a resource, using a POST endpoint for extensive parameter usage may make more sense. This allows us to send the entire body of the message to the API.
We could design it as a resource instead of sending a GET request to a resource with multiple parameters in the query string, which may result in a tremendously long un-debuggable URL. We could even utilize this to cache our computation results, depending on what our API has to do to fulfill our request.
We'd POST a new request to our /searches endpoint, with the body including our search configuration/parameters. We get a search ID, which we can use to GET our search results later.
Conclusion
As with other best practices, our role as API designers is to figure out how our APIs are used, not to follow one technique as "the best option." The most common use cases should be the easiest to complete, and it should be extremely impossible for a user to make a mistake.
When getting data through APIs, query string parameters are helpful. Using the correct query strings can limit the number of responses provided in the most simple circumstances, while others can incorporate several tables and databases with a single endpoint. The possibilities are virtually limitless.
As APIs become more sophisticated, a well-designed framework is required to make their use easier. The ability to filter and sort data using parameters, especially query string parameters, will only improve the API and give developers more tools. Finally, don't forget to read the documentation.
Atatus API Monitoring and Observability
Atatus provides Powerful API Observability to help you debug and prevent API issues. It monitors the consumer experience and be notified when abnormalities or issues arise. You can deeply understand who is using your APIs, how they are used, and the payloads they are sending.
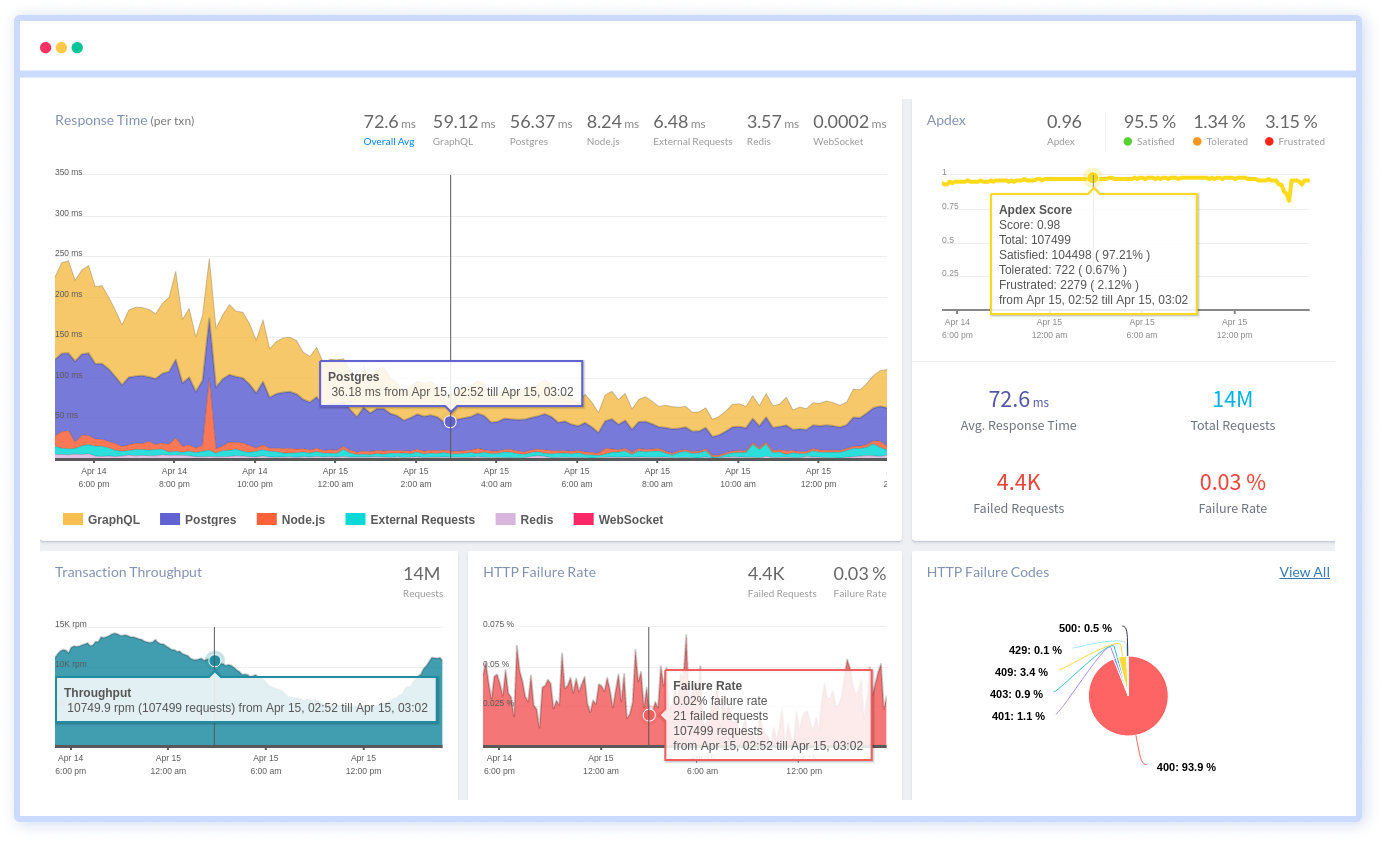
Atatus's user-centric API observability tracks how your actual customers experience your APIs and applications. Customers may easily get metrics on their quota usage, SLAs, and more.
It monitors the functionality, availability, and performance data of your internal, external, and third-party APIs to see how your actual users interact with the API in your application. It also validates rest APIs and keeps track of metrics like latency, response time, and other performance indicators to ensure your application runs smoothly.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More