Back in the day, frontend development was all about writing CSS, HTML, and JavaScript. However, it is no more the case. Now it has become much more complex and interesting than earlier. In addition, the e-commerce industry is evolving continuously, making frontend developers stay at par with the latest frontend technologies to create and build efficient and highly optimized websites for their businesses.
In today’s article, we will see a definitive guide to SCSS, what it means and how to use it. We shall also see SCSS’s application in the real world and the difference between SCSS and Sass.
Table Of Contents
- Introduction to SCSS
- SCSS vs Sass: The key difference
- Why do you need SCSS?
- SCSS language syntax
- SCSS Comments
- SCSS Nesting
- SCSS Nesting using &
- Variables
- Mixins
- Importing SCSS Stylesheet (@import)
#1 Introduction to SCSS
SCSS, which stands for Sassy-CSS or Sassy Cascading Style Sheets, is also officially described and known as “CSS with superpowers”. SCSS is a well-known preprocessor tool through which a developer can write styles for their websites with more interesting and advanced CSS syntax.
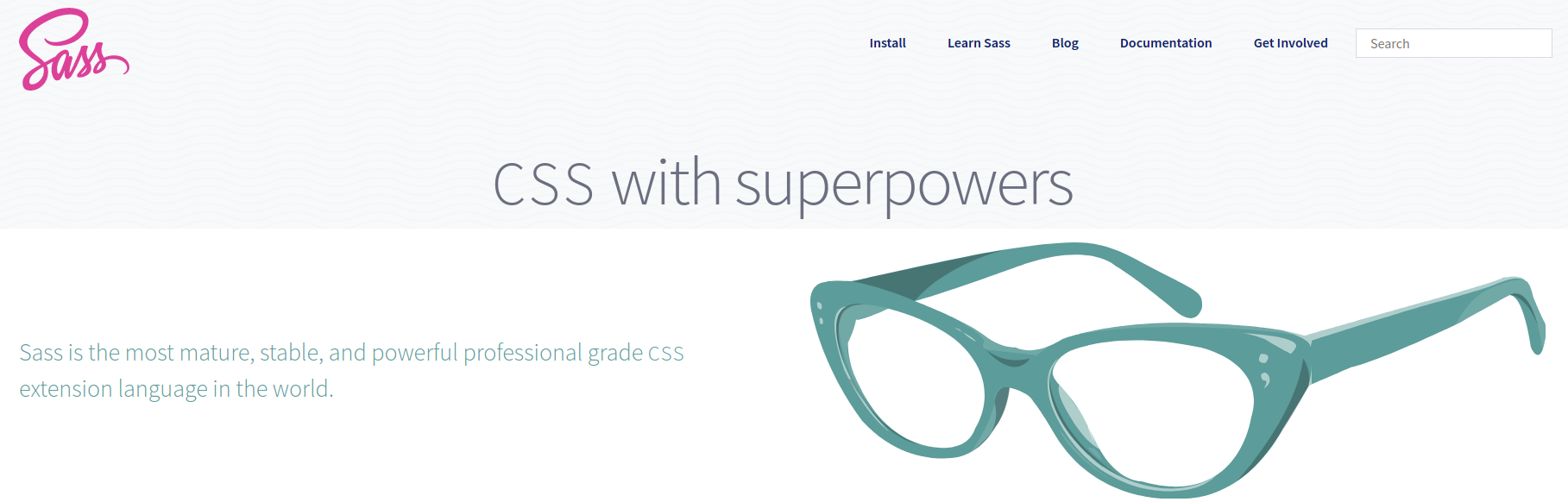
In general, browsers are not functioned to process or understand Sass/ SCSS features or codes, such as mixins, functions, and nesting. Therefore, to run them in a browser, one needs to convert them to a regular CSS file.
#2 SCSS vs Sass: The key difference
Most beginners and developers get confused between these two terms: SCSS and Sass. Although SCSS is derived from and is similar to Sass, both of them have different syntax flavours. The key difference between SCSS and Sass is their syntax (the way developers write them).
As discussed, SCSS stands for Sassy CSS, and its file is written with the .scss extension that follows the traditional curly braces, semicolon, and block-like structure with the braces { } to write the extension.
On the contrary, Sass is an older syntax (known as the indented syntax), whose file is written with the .sass extension, that uses the strict indentation based structure with no semicolons, similar to Python syntax.
Example of SCSS:
$font-stack: Helvetica, sans-serif;
$primary-color: #333;
body {
font: 100% $font-stack;
color: $primary-color;
}
Example of Sass:
$font-stack: Helvetica, sans-serif
$primary-color: #333
body
font: 100% $font-stack
color: $primary-color
The above example will produce the following css:
body {
font: 100% Helvetica, sans-serif;
color: #333;
}
Whether you choose Sass or SCSS, you can easily convert one to the other with the help of the sass-convert tool. In addition, SCSS’s features and new syntax reduces the amount of time required to write a CSS rule.
#3 Why do you need SCSS?
CSS is cool and useful but not useful enough. SCSS was created to make developer’s lives easy; hence this entire new language was introduced to them. Originally, Sass was created for this purpose, and SCSS is its enhanced, improved, and much-advanced version.
#4 SCSS language syntax
For the syntax of the SCSS language, we shall introduce its structure and some common and basic syntax used within the language by the developers.
SCSS follows a similar structure to that of CSS. It begins with choosing one or multiple elements using classes, IDs, or other CSS selectors and then adding styles. SCSS supports all of the CSS properties.
Let us have a look at the example below:
.gist {
.gist-file {
border: none;
border-bottom: none;
}
.gist-meta {
background: #ccc;
border-radius: 0 0 4px 4px;
font-size: 14px;
padding: 6px 16px;
a {
color: #E96B2C;
&:hover {
text-decoration: underline;
}
}
}
}
#5 SCSS Comments
SCSS allows both multi-line (/* */) and single-line (//).
The examples for both are as below,
// This is the example of a single-line comment allowed in SCSS
/* This is the example of a multi-line comment allowed in SCSS */
#6 SCSS Nesting
Nesting in SCSS is much more organized and simpler than the CSS.
Lets take navbar styles
.navbar i {
color: white;
}
.navbar ul {
padding: 0px;
}
.navbar a {
display: block;
padding: 10px 0px 10px 25px !important;
}
.navbar a:hover {
background: white !important;
color: black !important;
}
.navbar a:hover i {
color: black;
}
But now, this can be written in SCSS as:
.navbar {
i {
color: white;
}
ul {
padding: 0px;
}
a {
display: block;
padding: 10px 0px 10px 25px !important;
&:hover {
background: white !important;
color: black !important;
i {
color: black;
}
}
}
}
This concise and much-organized style has the following major advantages than the CSS:
- Since all the nav’s and its children’s styles are within the curly braces, one need not waste their time finding it in a file or multiple files.
- As there is no repetition, one writes a simpler and smaller size code.
However, one needs to be mindful of keeping the selectors shallow. It is because going much deeper into SCSS nesting can make the CSS files even larger, making the browser do more work in styling the elements. Thus, even though coding gets much simpler, organized, and concise through nesting, it is important to be mindful and careful not to overuse it, as it could lead to several problems.
#7 SCSS Nesting using &
Often in CSS, some styles are the extended versions of other styles. For example, if in CSS a code is written like this:
header {
width: 40%;
margin: 0 auto;
}
header-link {
color: red;
}
You can write a similar code in SCSS with much more ease and simplicity using & character in nesting.
header {
width: 40%;
margin: 0 auto;
}
&-link {
color: red;
}
SCSS is a preprocessor that reads the second example and compiles that example within the first example. The name of the current selector gets replaced with the & symbol. Thus, one can nest selectors as deep as required. However, it is recommended to usually go up to three levels of nesting using &.
#8 Variables
Variables are used to store data. One can save and set any CSS value to a variable, including units in the .scss file. The variables are denoted using the $ symbol.
The syntax for declaring variables in SCSS is:
$font-text: 'Open Sans', 'san-serif', 'Noto Sans';
$font-color: #29a634;
$width-container: 700px;
One can call the variables defined above in the .scss style as:
.container {
width: $width-container;
font-family: $font-text;
color: $font-color;
}
SCSS variables are very beneficial and useful to keep colours, fonts, and other values consistent throughout a web app or a website. Another crucial benefit of using variables in SCSS is that just by changing the variable’s value, they can alter or change every selector that uses those variables.
When it comes to SCSS variables, one needs to keep the following things into consideration:
- Every variable defined at the top level is global.
- Every variable defined within the blocks or the curly brackets is local.
- A block can assess both the global and local variables.
For example,
$global-variable: "I am a global variable";
div {
$local-variable: "I am a local variable";
}
#9 Mixins
Through a mixin, one can declare multiple CSS styles that can be reused, meaning it is a reusable group of CSS declarations. Mixins have similar syntax as that functions in JavaScript. Therefore, we use a @mixin directive instead of the functions keyword.
In addition, you can call a mixin using the @include statement.
To centre everything in a flex box using mixin as below:
@mixin center-position()
{
display: flex;
justify-content: center;
align-items: center;
}
This mixin is called in the .scss file, whenever required as below:
.centered-container {
@include center-position();
}
.centered-container-red {
@include center-position();
background: $color-red;
}
#10 Importing SCSS Stylesheet (@import)
While creating larger applications, chunking a code is considered the best practice. By creating a main.scss file, one can import other .scss files into the parent file.
@import './styles.scss';
@import './variables.scss’;
Conclusion
SCSS allows web developers to use variables, mixins, and imports together to write reusable styles in an organized manner. Going through the SASS documentation is recommended to get a much deeper insight and get an even wider understanding of SCSS and its features.
Thus, SCSS helps develop particular naming patterns and adding a reusable element into a code. Once you are thorough and comfortable using SCSS, it would also help to go through and understand the built-in SCSS modules, which can help you while writing larger codes for complex applications.