What is JavaScript localStorage - A Complete Guide for Beginners
For basic applications that don't require a server, the localStorage API provides a powerful built-in function that eliminates the need for databases. We can use this API to save data locally so that our application can access it.
We'll go through how to use the localStorage function in JavaScript in this article.
We will go over the following:
- What is JavaScript localStorage?
- localStorage vs. Cookies
- localStorage vs. sessionStorage
- Where is localStorage Stored?
- What is Window.localStorage?
- localStorage Methods
- localStorage Browser Compatibility
- Advantage of localStorage
- Limitations of localStorage
What is JavaScript localStorage?
localStorage is a sort of web storage that stores data. This allows JavaScript sites and applications to store and retrieve data without having to worry about it expiring. This ensures that data will never be lost and will never expire. As a result, data saved in the browser will remain accessible even after the browser window has been closed.
The storage API is beneficial since it eliminates the need to send all of a user's session data to a database. You can track if a user has enabled dark mode on your site on the client-side. You don't need to save a half-completed form in your database if a user saves it for later.
In a nutshell, localStorage stores data that has no expiration date and is accessible to the user even after the browser window has been closed. It can be used in a variety of ways, such as remembering the data of a shopping cart or sign in to a website.
Cookies used to be the only way to remember this type of temporary and local information, but now we now have localStorage. Cookies have a lower storage limit than local storage. It isn't delivered with every HTTP request, either. As a result, it is now a better option for client-side storage.
localStorage vs. Cookies
For starters, unlike cookies, data kept in localStorage is not transferred to the server with every request. As a result, you'll be able to store more data in the localStorage.
The localStorage feature of most modern web browsers allows you to save up to 5MB of data. It's worth noting that cookies can hold up to 4KB of data.
Second, the client, specifically JavaScript in the web browser, can manage the data stored in localStorage. The servers are unable to access it. Cookies, on the other hand, can be handled by JavaScript in both web browsers and servers.
localStorage vs. sessionStorage
Web storage can be classified into two parts. localStorage refers to data that remains in a user's browser window after they close it. localStorage data, unlike cookies, has no expiration date. sessionStorage is data that lasts until the browser is closed, or until a session expires.
When compared to cookies, both localStorage and sessionStorage have advantages:
- The data is only saved locally and cannot be viewed by the server, therefore they are no longer a security risk that cookies present
- It helps you to save a lot more data (10Mb for most browsers)
- It's a lot easier to use, and the syntax is quite simple
It's also compatible with all modern browsers, so you may use it right now. Cookies still have a use, especially when it comes to authentication because the data can't be accessed on the server.
localStorage and sessionStorage perform the same thing and have the same API, but sessionStorage persists data just until the window or tab is closed, but localStorage persists data until the user clears the browser cache manually or until your web application clears the data.
Where is localStorage Stored?
Web storage data in Google Chrome is saved in an SQLite file in a subfolder in the user's profile.
- \AppData\Local\Google\Chrome\User Data\Default\ Local Storage on Windows
- /Library/Application Support/Google/Chrome/Default/Local Storage on macOS
Firefox saves storage objects in the webappsstore.sqlite (SQLite file), which is also in the user's profile folder.
How to View localStorage Data?
Many browser extensions save their data in the browser's so-called Local Storage, which is just a storage location controlled by the web browser. Everything is saved locally on the machine where the browser is installed, as the name implies. The cloud does not include local storage.
Here's how to view the data:
Go to Chrome's extension page first. Open the background page for the extension you want to examine. This may be done by clicking the "Inspect views: background page" link in each extension.
Simply hit F12 to launch the developer tools, then select the Application tab when the extension's background page is visible. After that, expand Local Storage in the Storage section. Following that, you'll be able to examine all of your browser's local storage.
What is window.localStorage?
The window interface's localStorage read-only feature allows you to access a Storage object for the Document's origin; the data is saved throughout browser sessions.
While localStorage data does not have an expiration date, sessionStorage data is cleared when the page session expires — that is, when the page is closed. But when the last "private" tab is closed, the localStorage data for a page loaded in a "private browsing" or "incognito" session is removed.
Syntax:
myStorgae = window.localStorage;
localStorage always stores keys and values in the UTF-16 DOMString format, which takes two bytes per character. Integer keys, like objects, are automatically converted to strings. The data in localStorage is particular to the document's protocol. localStorage returns a distinct object for a site loaded over HTTP than localStorage for the comparable site loaded over HTTPS.
LocalStorage appears to return a separate object for each file: URL in all current browsers. To put it another way, each file: URL appears to have its own local storage space. However, there are no certainties about this behaviour, therefore you shouldn't count on it because, as previously said, the requirements for file: URLs are yet unknown.
As a result, browsers may change their localStorage file: URL handling at any time. In reality, throughout time, some browsers' handling of it has evolved.
localStorage Methods
Some methods for using localStorage are provided. We'll go over each of these localStorage methods. Before we get into that, here's a quick rundown of the methods:
- setItem(): This method is used to add data to localStorage using key and value
- getItem(): This method is used to fetch or retrieve the value from the storage using the key
- removeItem(): It uses the key to remove an item from storage.
- clear(): It's used to clear out all of the storage.
- key(): Takes a number as an argument and returns the key of a localStorage
setItem()
This method, as its name suggests, allows you to store values in the localStorage object. It requires two inputs such as a key and a value. The value associated with the key can be retrieved later by referencing the key.
window.localStorage.setItem('name', 'Joe');
window.localStorage.setItem('age', 10);
window.localStorage.setItem('user', '{"name": "Justin"}');
The key is name, and the value is Justin. It's also worth mentioning that localStorage can only hold strings. You'd have to convert arrays or objects to strings to store them.
getItem()
Use the getItem() function to retrieve items from localStorage. You can use getItem() to get data from the browser's localStorage object.getItem() takes only one parameter, the key, and returns a string as the value.
To get a user key, do the following:
window.localStorage.getItem('user');
This produces a string with the following value:
{"name":"Justin"}
removeItem()
Use the removeItem() method to destroy local storage sessions. When provided a key name, the removeItem() method removes that key from the storage if it exists. This function will do nothing if there is no object linked with the specified key.
window.localStorage.removeItem('user');
clear()
To erase all things in localStorage, use the clear() function. When this method is called, the full storage of all records for that domain is cleared. There are no parameters sent to it.
window.localStorage.clear();
key()
When you need to loop through keys, the key() function comes in handy because it allows you to pass a number or index to localStorage to get the name of the key.
var KeyName = window.localStorage.key(index);
localStorage Browser Compatibility
HTML 5 specifies localStorage, which is supported by a number of browsers, including Chrome. Below is a list of browsers that support JavaScript localStorage, along with respective versions.
- Google Chrome – 4.0
- Internet Explorer – 8.0
- Firefox – 3.5
- Opera – 11.5
- Safari – 4
- Edge – 12
You may test browser compatibility with the help of the code below. Before adding or deleting something from localStorage, use this code in every localStorage program to check browser compatibility.
<script>
// Code to check browser support
if (typeof(window.localStorage) !== "undefined") {
// browser supports local storage
} else {
// browser does not support local storage
}
</script>
Advantage of localStorage
There are various advantages of using localStorage. The first and most important benefit of localStorage is that it can store temporary but relevant data in the browser that persists even after the browser window has been closed. The following is a list of some of the benefits:
- The data that localStorage collects is stored in the browser. In the browser, you can save up to 5 MB of data
- Data stored by localStorage has no expiration date
- With a single line of code, you may clear all of the localStorage items
- localStorage data persists long when the browser window is closed
- It also has an advantage over cookies in that it can store more data
Limitations of localStorage
Since localStorage allows you to store temporary, local data that persists even after you close the browser window, it has a few drawbacks. Some of localStorage's limitations are listed below:
- localStorage should not be used to store sensitive information such as usernames and passwords
- Data is not protected in localStorage, and it can be accessed with any code. As a result, it's highly risky
- Using localStorage, you can only store up to 5MB of data on the browser
- localStorage simply stores data in the browser, not in a server-based database
- Each operation in localStorage is synchronous, which means they all happen at the same time
Summary
The localStorage method in JavaScript allows you to save data locally. It's significantly better than utilizing a database for simple applications like a to-do list, saving user preferences, or saving a user's form data (without including sensitive data). localStorage is simple to set up, use and is made entirely of vanilla JavaScript.
You're now ready to use the localStorage method. Don't forget to monitor JavaScript applications for better performance.
Monitor Your JavaScript Applications with Atatus
Atatus keeps track of your JavaScript application to give you a complete picture of your clients' end-user experience. You can determine the source of delayed load times, route changes, and other issues by identifying frontend performance bottlenecks for each page request.
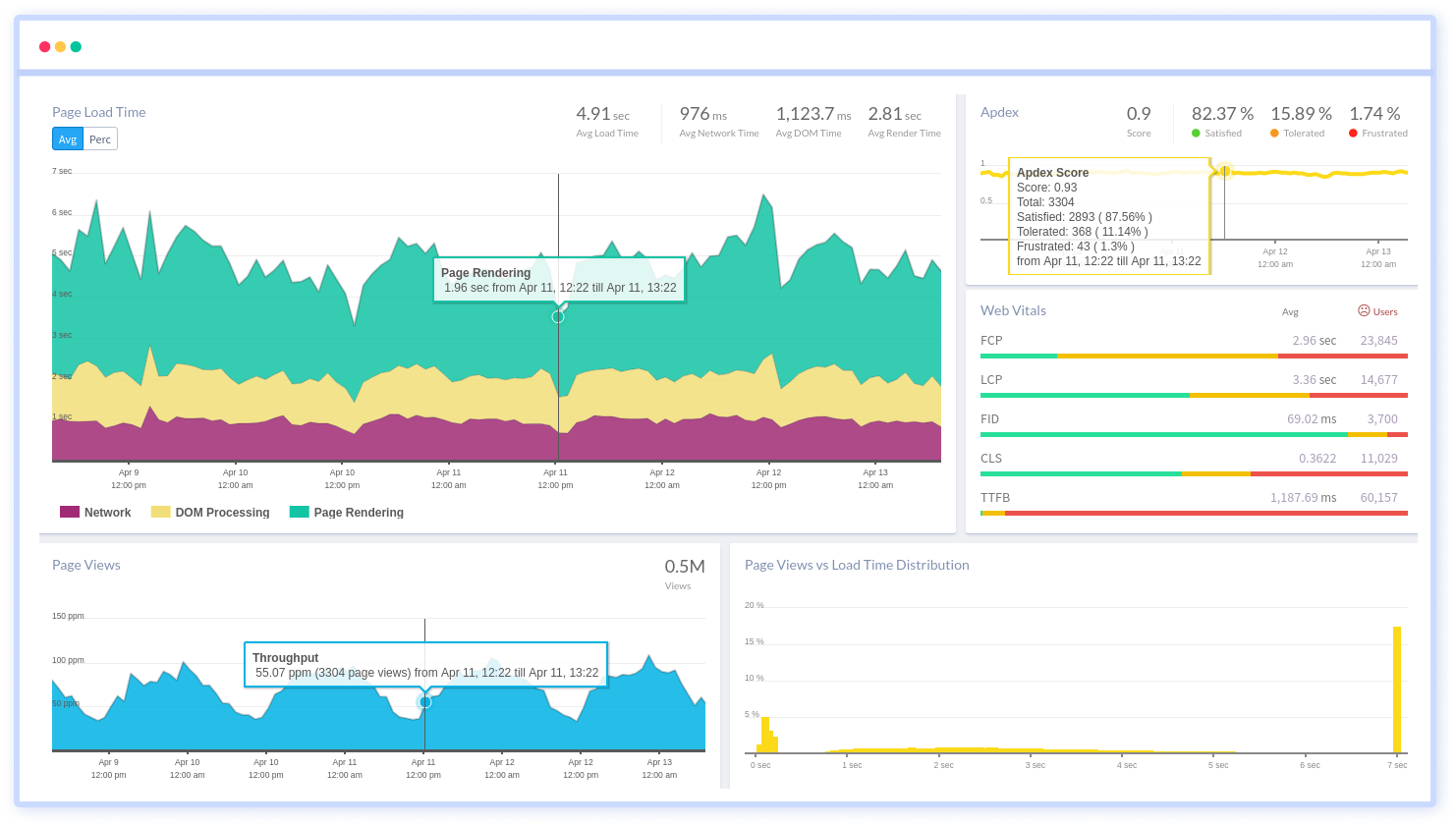
To make bug fixing easier, every JavaScript error is captured with a full stack trace and the specific line of source code marked. To assist you in resolving the JavaScript error, look at the user activities, console logs, and all JavaScript events that occurred at the moment. Error and exception alerts can be sent by email, Slack, PagerDuty, or webhooks.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More