How to Log to Console in PHP and Why Should You Do It
Monitoring, troubleshooting, and debugging your code all require logging. It not only makes the underlying execution of your project more visible and understandable, but it also makes the approach more approachable. Intelligent logging procedures can assist everyone in a company or community to stay on the same page about the project's status and progress.
PHP is one of the most popular server-side scripting languages for developing web applications due to its ease of use. Making it simple to track errors is critical to guaranteeing a quick code-test-learn feedback loop, no matter what you're building.
Even though the browser is the most common way to interact with websites and web applications, and PHP was specifically designed to be good for web application development, PHP didn't have an easy way to log errors to the browser console because it was created before today's modern browsers.
In this article, we'll show you how to log to the console in PHP, why it's a good idea to do console log in PHP, and how it's just as simple as logging to the console with JavaScript. So you can enjoy the power and simplicity of a powerful web programming language like PHP while still logging into the console within PHP.
We will cover the following:
- Logging in PHP
- What is Browser Console?
- Why Should You Log to the Console?
- How to Use PHP Code to Log Directly to the Console?
- Benefits of Logging to Console
Logging in PHP
PHP is still one of the most commonly used programming languages in the world, accounting for over 78 percent of the known internet, more than 25 years after its creation.
When we first started programming in PHP, we quickly realized that our Javascript background had not equipped me for this. Working with PHP's native logging capabilities couldn't fill that hole because we were so used to troubleshooting with the web console. But there was reason to be optimistic!
Before we get into it, let's have a look at how PHP handles logging and debugging.
Logging in PHP can be accomplished in two ways:
- By writing to log files on the hard drive
- Using echo and var_dump to display variable values on web pages
Both of these alternatives have the potential to be constrictive.
Static log files can help you analyze how the execution went in the past, but they can also deprive you of the wonderful real-time experience of watching the dynamics of a modern website teeming with network requests and socket connections.
Also, you can only put so much content on your website before it starts to look cluttered. As a result, traditional logging systems available in PHP can appear less practical for larger, dynamic websites.
The aforementioned concerns limit logging capabilities not only for PHP but for any future web development language.
What is Browser Console?
As the name implies, the browser console is a console within the browser.
The console in your browser allows you to run Javascript code and log information relevant to your website, just like you can run Python or Node.js code on a console in your terminal and display results and log errors. Network requests, JavaScript, CSS, security errors, and warnings, as well as explicit error, warning, and informational messages logged by JavaScript code running in the page context, are all logged.
We'll use the desktop version of Google Chrome for example, but equivalent processes can be performed in desktop versions of Firefox, Safari, and Internet Explorer.
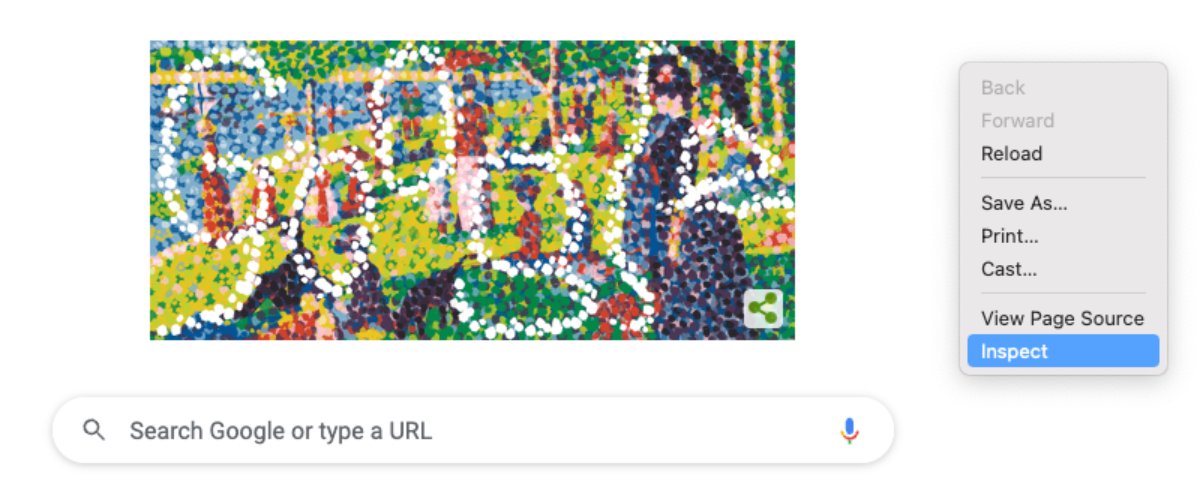
To begin, open Google Chrome and navigate to any web page. Right-click the page and select Inspect to access Chrome's Developer Tools.
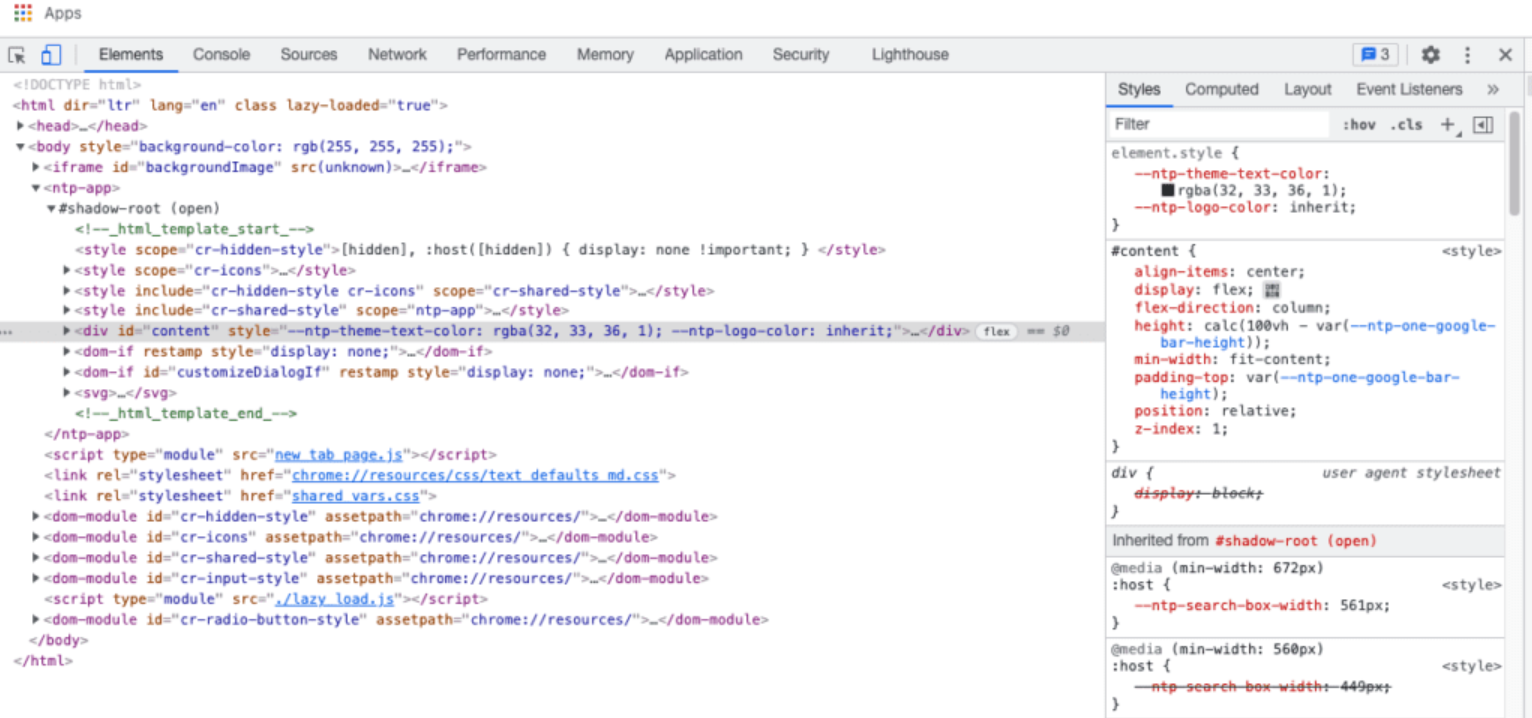
One of the tabs in the Developer Tools will be the browser console. You can also test it by using the same console.log command in JavaScript.
Let's imagine you built an online messaging platform and people on your website were unable to receive messages from their buddies for some reason. In this scenario, having a clear recording system in place would be beneficial in determining what went wrong and where it occurred.
Here's an example of a log:
- Database connection
- Opening socket connection
- Successful socket connection
- Fetching user’s friends
- Processing data
- Rendering data
- Sending a message on the channel
- Received a message on the channel
- Rendering message
- Error in rendering message
As seen in the previous example, properly configured logs can assist you in determining that, while the message content was received, your code failed to present it. You can now move to the relevant section of your code and make the necessary modifications.
Why Should You Log to the Console?
You need to do a console log for two reasons:
- Simplicity – You should only use two applications as a PHP developer: code editor or IDE and browser. As you write code in the editor and test it in the browser, you switch between the two. The browser is the most obvious place to display log statements.
- To Keep the Logging as Least Intrusive as Possible – You can now use native PHP functions like var_dump to log. When using var_dump, however, you must first determine where you want the output to be written. It could be the web page in the browser, but this will most certainly distort the display. A file on your server is another option for output, however, we did recommend using an open-source logging library like monolog instead of var_dump for this. If you want to output view variables (variables that are primarily connected to display) without distorting the web page, logging into the browser console is a preferable option.
Another thing to keep in mind is that PHP developers are increasingly gravitating toward frameworks like Laravel and Symfony, which use popular PHP logging libraries like monolog. These libraries are most useful when you need a full explanation of the error stack trace for server-side issues such as database connection and file output.
For front-end debugging, you might just want something simple to display inside the browser. Logging to the console would be good in these cases. Additionally, for a more full development setup, you can combine this technique with regular PHP logging mechanisms.
How to Use PHP Code to Log Directly to the Console?
You can log straight to the console using PHP code in three methods. In a nutshell, we used the json_encode() function and PHP libraries.
#1 Using json_encode() Function
Let's say you wish to log a PHP variable named $view_variable in your view layer to the console. Console.log() is a JavaScript function, as you may recall. The important concept is that we can transmit the PHP variable to the JavaScript code using JSON. You write a PHP function that looks like this:
<?php
function console_log($output, $with_script_tags = true)
{
$js_code = 'console.log(' . json_encode($output, JSON_HEX_TAG) . ');';
if ($with_script_tags)
{
$js_code = '<script>' . $js_code . '</script>';
}
echo $js_code;
}
As shown above, you can call this function at the exact location where you want to run the console_log() that we just constructed. An example of its application would be as follows:
<?php $view_variable = 'a string here'; ?>
<!-- HTML content -->
<div>
<!-- HTML content -->
</div>
<?=console_log($view_variable); ?>
And the resulting HTML markup would be as follows:
<!-- HTML content -->
<div>
<!-- HTML content -->
</div>
<script>console_log('a string');</script>
You can call the modified console log PHP function as many times as you need as long as you remember to include the definition. You can refer to the list of constants used by json_encode() if you want to translate the JSON string into another format. JSON_FORCE_OBJECT and JSON_PRETTY_PRINT are two useful constants that you're more likely to employ.
#2 In the Middle of Your JavaScript Code
You should only install PHP libraries if you are 100% certain you need them. As a result, you might prefer to console log the PHP variables in the middle of your PHP view files' JavaScript code. You may apply the same technique in the following way:
<script>
// JavaScript code ...
var js_variable_as_placeholder = <? = json_encode($view_variable, JSON_HEX_TAG); ?>;
console.log('a string');
// JavaScript code ...
</script>
#3 Using PHP Libraries to Console Log
There are two choices we recommend if you prefer to use open-source libraries that have addressed this issue. They are PHPDebugConsole and PHPConsole.
PHPDebugConsole vs. PHPConsole
The following analysis is based on a quick comparison of the two libraries.
With 1,343 stars on GitHub, PHPConsole appears to be more established, while PHPDebugConsole has 50 stars. Since late 2018, PHPConsole hasn't been updated, and it has fewer commits than PHPDebugConsole.
In January 2019, PHPDebugConsole has a more frequent updating history. In addition, PHPDebugConsole offers greater documentation. Their documentation contains demo examples and expressly recommends numerous browser extensions with which it can function.
On the other side, PHPConsole suggests a Chrome Extension that appears to be created by the same individuals that created PHPConsole.
In light of the foregoing, if you want to use a PHP library to log console messages, we strongly suggest PHPDebugConsole. In general, we would suggest the following choice matrix:
- Use the PHP json_encode function if you want to keep things simple
- Use PHPDebugConsole with PHPConsole as a backup alternative if you want to use more advanced capabilities like console.info()
On their website, they provide a usage example that demonstrates how simple it is to get started with logging in using PHPDebugConsole.
<?php
require __DIR__ . '/vendor/autoload.php';
$debug = new \bdk\Debug(array(
'collect' => true,
'output' => true,
));
$debug->log('hello world');
An example of HTML output from their website is seen below.
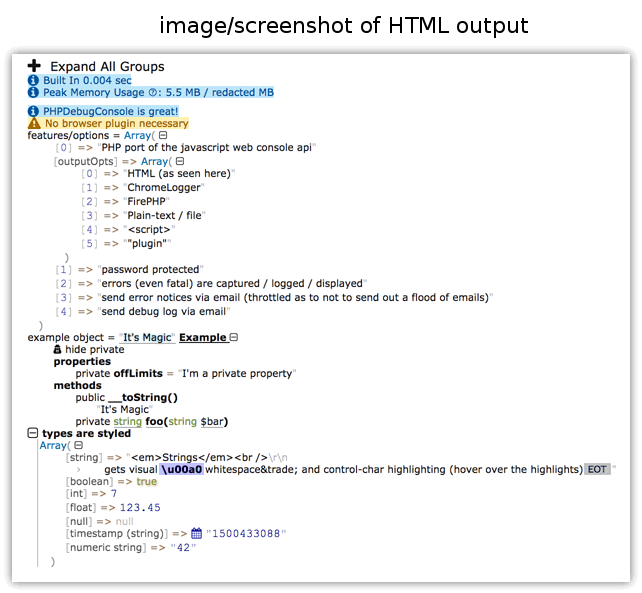
By installing certain extensions, they also support logging into the browser's console.
Nothing beats the simplicity of using your custom json_encode() based helper function if you're working on a small-scale personal project, in my opinion. It would relieve you of the burden of managing external dependencies and browser compatibility.
Benefits of Logging to Console
The following are the benefits of logging into the console:
- Convenience
Logging into the browser console is unquestionably more convenient. It is more convenient for a developer to have the mistakes logged immediately next to the display of the web page. Instead of having a log file open on the side that is less likely to be auto-refreshed, the developer merely has to move between the IDE and the browser. - Doesn't Cause Any Issues with the Website
The PHP functions echo and var_dump can be used to console log and monitor data on a web page. However, as we previously noted, it causes display issues, degrades the user experience, and is not a smart approach for large projects. Since the console logs can be displayed in a separate browser console window, developers don't have to worry about altering the web page layout or the formatting of their logs. - Real-time Monitoring of Results
Since the display and content rendering of a web page is such an important part of a website's functionality, the developers must be able to monitor the logs and the website's performance in real-time as the web page unfolds and the user interacts with it. This synchrony can open up new perspectives on how the website is operating and what adjustments might be done. - Saves You the Trouble of Going through Large Static Log Files
Even while it is often necessary to track and maintain log files for large-scale projects to analyze and assess performance, it can be discouraging to learn that PHP is the language's only logging capability. It can be useful to have a console that displays results as and when needed for less important debugging logs.
Summary
Web programming is at a very intriguing and exciting period right now. Previously, the console_log() could only be utilized with JavaScript. You learned what the console_log() is, why it's useful for web development, and how to log PHP variables on your browser console with your PHP code in this article.
If you require more advanced features, such as console.info(), PHP libraries such as PHPDebugConsole can assist you. We highly suggest using monolog to cover more back-end-related debugging needs to make it a complete, end-to-end logging infrastructure for your PHP web application.
Atatus: PHP Performance Monitoring and Log Management
Atatus is an Application Performance Management (APM) solution that collects all requests to your PHP applications without requiring you to change your source code. However, the tool does more than just keep track of your application's performance. It monitors logs from all of your PHP applications and systems into a centralized and easy-to-navigate user interface, allowing you to troubleshoot faster.
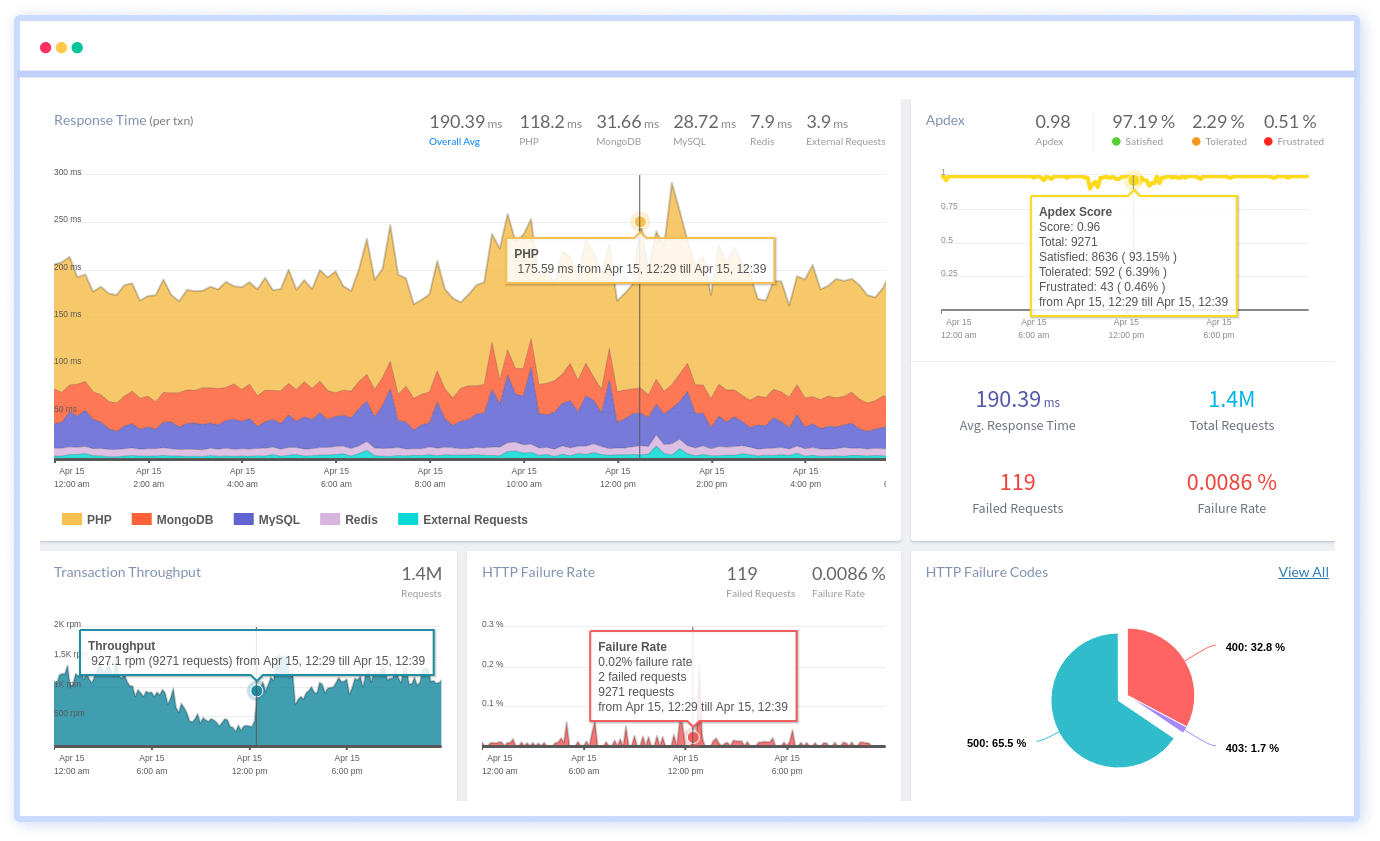
We give a cost-effective, scalable method to centralized PHP logging, so you can obtain total insight across your complex architecture. To cut through the noise and focus on the key events that matter, you can search the logs by hostname, service, source, messages, and more. When you can correlate log events with APM slow traces and errors, troubleshooting becomes easy.
We offer Application Performance Monitoring, Real User Monitoring, Serverless Monitoring, Logs Monitoring, Synthetic Monitoring, Uptime Monitoring, and API Analytics. It works perfectly with any application, regardless of framework, and has plugins.
#1 Solution for Logs, Traces & Metrics
APM
Kubernetes
Logs
Synthetics
RUM
Serverless
Security
More